Converting a Python string to an integer and vice versa.
In this tutorial, we will be taught the process of converting python strings to integers and integers to strings in python. In our prior tutorial, we comprehended the concept of the append function in Python lists.
Convert a Python string into an integer.
If you have read our previous tutorials, you might have noticed that we often require this conversion. It is frequently necessary, such as when you are retrieving data from a file and it is in the form of a String, and you need to convert it to an int. Now, let’s proceed directly to the code. To convert a string representation of a number to an int, you need to utilize the int() function. Consider the following example:
num = '123' # string data
# print the type
print('Type of num is :', type(num))
# convert using int()
num = int(num)
# print the type again
print('Now, type of num is :', type(num))
The result of the code will be.
Type of num is : <class 'str'>
Now, type of num is : <class 'int'>
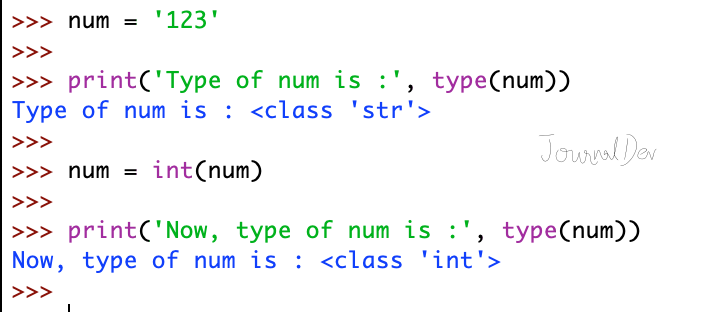
Converting a String to an int with varying bases.
If the string you want to convert to an integer originates from a number system other than base 10, you can indicate the base for the conversion. However, it is important to note that the resulting integer will always be in base 10. Additionally, the specified base must be within the range of 2 to 36. To grasp the conversion of a string to an integer using the base argument, refer to the following example.
num = '123'
# print the original string
print('The original string :', num)
# considering '123' be in base 10, convert it to base 10
print('Base 10 to base 10:', int(num))
# considering '123' be in base 8, convert it to base 10
print('Base 8 to base 10 :', int(num, base=8))
# considering '123' be in base 6, convert it to base 10
print('Base 6 to base 10 :', int(num, base=6))

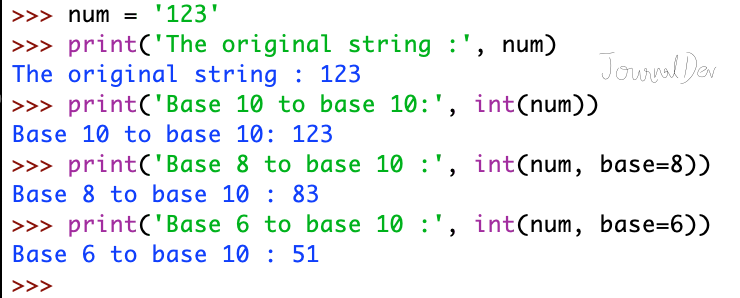
An error occurs while attempting to convert a string to an integer.
When converting from a string to an integer, you might encounter a ValueError exception. This exception occurs if the string you are trying to convert does not contain any numbers. For instance, if you are trying to convert a hexadecimal number to an integer but forget to specify the base as 16 in the int() function, a ValueError exception will be raised if any of the digits in the string do not belong to the decimal number system. The upcoming example will demonstrate this exception when converting a string to an integer.
"""
Scenario 1: The interpreter will not raise any exception but you get wrong data
"""
num = '12' # this is a hexadecimal value
# the variable is considered as decimal value during conversion
print('The value is :', int(num))
# the variable is considered as hexadecimal value during conversion
print('Actual value is :', int(num, base=16))
"""
Scenario 2: The interpreter will raise ValueError exception
"""
num = '1e' # this is a hexadecimal value
# the variable is considered as hexadecimal value during conversion
print('Actual value of \'1e\' is :', int(num, base=16))
# the variable is considered as decimal value during conversion
print('The value is :', int(num)) # this will raise exception
The above code will produce the following result.
The value is : 12
Actual value is : 18
Actual value of '1e' is : 30
Traceback (most recent call last):
File "/home/imtiaz/Desktop/str2int_exception.py", line 22, in
print('The value is :', int(num)) # this will raise exception
ValueError: invalid literal for int() with base 10: '1e'
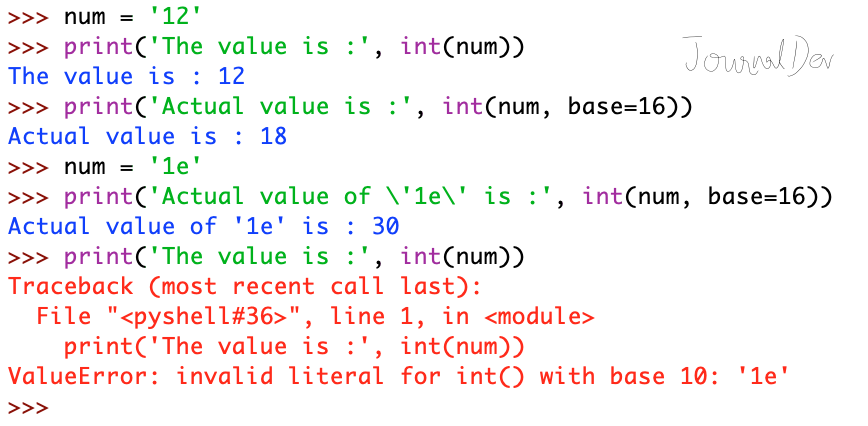
Converting an integer to a string in Python.
Converting an integer to a string is effortless and does not require any verification. You can simply utilize the str() function to carry out the conversion. Take a look at the subsequent example.
hexadecimalValue = 0x1eff
print('Type of hexadecimalValue :', type(hexadecimalValue))
hexadecimalValue = str(hexadecimalValue)
print('Type of hexadecimalValue now :', type(hexadecimalValue))
The result of running the given code will be:
Type of hexadecimalValue : <class 'int'>
Type of hexadecimalValue now : <class 'str'>
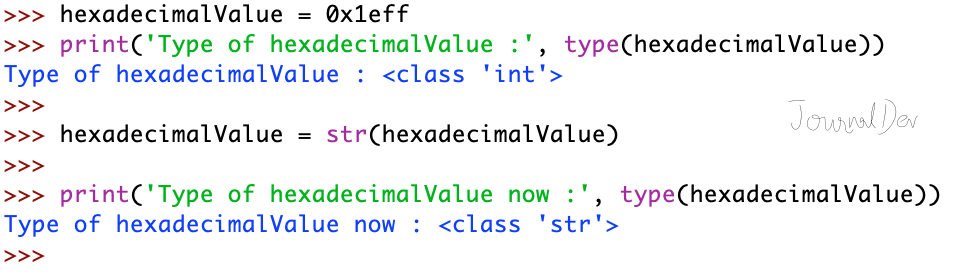
This concludes the discussion on converting a string to an integer and vice versa in Python. For more information, refer to the official Python documentation.
More Tutorials
convert string to character array in Java.(Opens in a new browser tab)
The Python functions ord() and chr()(Opens in a new browser tab)
Adding a string to a Python variable(Opens in a new browser tab)