Creating an Android Spinner by utilizing Kotlin.
In this tutorial, we will talk about and implement Spinners in our Android app using Kotlin. The Android Spinner is utilized to generate a dropdown menu on the display.
What are you going to be taught?
- Creating Spinners through XML and Programmatically
- Setting a prompt on the Spinner.
- Creating a custom layout for the Spinner.
- Handling Click Listeners and Display a Toast message.
- Preventing the Click Listener from being fired automatically for the first time.
What does Android Spinner refer to?
Spinners are similar to drop-down menus that contain a selection of items. Once a value is chosen, the Spinner reverts back to its default state with the selected value. However, starting from Android 3.0, it is no longer possible to display a prompt as the default state in the Spinner. Instead, the first item in the list is shown. A Spinner in Android functions similarly to a phone charger being connected to an electricity board using an adapter. The adapter allows the Spinner (phone) to be loaded with data, just like how it provides electricity to the phone. In this case, the adapter specifies both the data and the layout for the items to be loaded in the Spinner.
Events for Spinner Callback
The AdapterView.onItemSelectedListener interface is utilized for invoking the callbacks of the Spinner click event. It comprises of two methods:
- onItemSelected
- onNothingSelected
In the upcoming portion, we will establish a fresh Android Studio Project and integrate Spinners into our Application. We will modify the designs and gain proficiency in managing various situations.
Project in Kotlin for an Android Spinner.
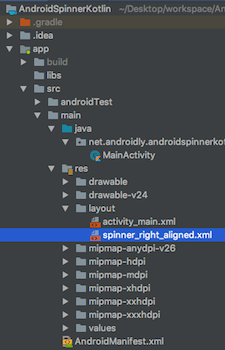
1. XML Code for Layout Design
Below is the code provided for the activity_main.xml layout file.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/linearLayout"
android:gravity="center"
tools:context=".MainActivity">
<Spinner
android:id="@+id/mySpinner"
android:layout_width="match_parent"
android:spinnerMode="dialog"
android:layout_height="wrap_content" />
</LinearLayout>
Currently, it holds only one Spinner where android:spinnerMode can either be set as dialog or dropdown.
To display prompts, set the spinnerMode value to dialog.
2. XML Code for a Spinner
Below is the code for spinner_right_aligned.xml.
<?xml version="1.0" encoding="utf-8"?>
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="end"
android:padding="15dp"
android:textAlignment="gravity"
android:textColor="@color/colorPrimary"
android:textSize="16sp"
/>
Option 1: Kotlin Code for the MainActivity
Here’s the provided code for the MainActivity.kt class.
package net.androidly.androidspinnerkotlin
import android.content.Context
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.view.Gravity
import android.view.View
import android.widget.*
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity(), AdapterView.OnItemSelectedListener {
var languages = arrayOf("Java", "PHP", "Kotlin", "Javascript", "Python", "Swift")
val NEW_SPINNER_ID = 1
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
var aa = ArrayAdapter(this, android.R.layout.simple_spinner_item, languages)
aa.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item)
with(mySpinner)
{
adapter = aa
setSelection(0, false)
onItemSelectedListener = this@MainActivity
prompt = "Select your favourite language"
gravity = Gravity.CENTER
}
val spinner = Spinner(this)
spinner.id = NEW_SPINNER_ID
val ll = LinearLayout.LayoutParams(LinearLayout.LayoutParams.MATCH_PARENT, LinearLayout.LayoutParams.WRAP_CONTENT)
ll.setMargins(10, 40, 10, 10)
linearLayout.addView(spinner)
aa = ArrayAdapter(this, R.layout.spinner_right_aligned, languages)
aa.setDropDownViewResource(R.layout.spinner_right_aligned)
with(spinner)
{
adapter = aa
setSelection(0, false)
onItemSelectedListener = this@MainActivity
layoutParams = ll
prompt = "Select your favourite language"
setPopupBackgroundResource(R.color.material_grey_600)
}
}
override fun onNothingSelected(parent: AdapterView<*>?) {
showToast(message = "Nothing selected")
}
override fun onItemSelected(parent: AdapterView<*>?, view: View?, position: Int, id: Long) {
when (view?.id) {
1 -> showToast(message = "Spinner 2 Position:${position} and language: ${languages[position]}")
else -> {
showToast(message = "Spinner 1 Position:${position} and language: ${languages[position]}")
}
}
}
private fun showToast(context: Context = applicationContext, message: String, duration: Int = Toast.LENGTH_LONG) {
Toast.makeText(context, message, duration).show()
}
}
Key ideas:
- Thanks to Kotlin Android extensions, the XML Spinner widget is automatically available in our Kotlin Activity class.
- We’ve created an arrayOf strings that consist of programming languages. These are filled in the adapter using the ArrayAdapter.
- The setDropDownViewResource is used to set the layout for the selected state and the spinner list rows.
- The android.R.layout.simple_spinner_item is used to set the default android SDK layout. By default, the TextView is left aligned in this type of layout.
We have now generated another Spinner using programming code that loads the layouts from the spinner_right_aligned.xml file.
To avoid the triggering of the OnItemSelected methods of the Spinner when the Activity is created, use setSelection(0, false).
– How does it function? The Activity is informed that the initial spinner item has been selected by the setSelection() method. This statement must be placed before onItemSelectedListener = this. To set the background color of the dropdown list, setPopupBackgroundResource is utilized. In the onItemSelected function, a Toast is triggered for the corresponding spinner item using the when statement. Thanks to Kotlin and functions with default values, we have minimized the lengthy Toast call.
The output of the Kotlin app for Spinner.
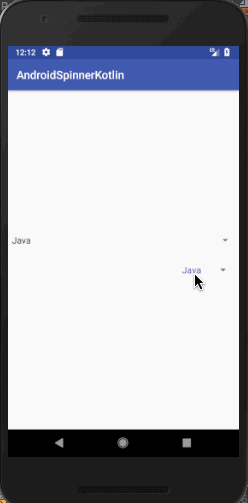
More tutorials
Creating an Android ProgressBar utilizing Kotlin.(Opens in a new browser tab)
Using Kotlin Android SharedPreferences(Opens in a new browser tab)
A class in Kotlin that is sealed.(Opens in a new browser tab)
One example of Spring REST XML and JSON(Opens in a new browser tab)
Convert string to XML document in Java(Opens in a new browser tab)