How to Delete Elements from an Array in Java
In Java, when we declare an array, we mention its data type and size. The JVM uses this information to allocate the required memory for the elements in the array. However, there are no dedicated methods available to delete elements from an array.
Removing an element from an array by utilizing a for loop.
To employ this approach, it is necessary to generate a fresh array. By utilizing a for loop, we can populate the new array while excluding the element we intend to remove.
package com.scdev.java;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = new int[]{1,2,3,4,5};
int[] arr_new = new int[arr.length-1];
int j=3;
for(int i=0, k=0;i<arr.length;i++){
if(i!=j){
arr_new[k]=arr[i];
k++;
}
}
System.out.println("Before deletion :" + Arrays.toString(arr));
System.out.println("After deletion :" + Arrays.toString(arr_new));
}
}
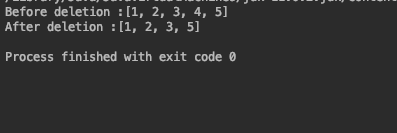
The code deletes the item at index 3. This approach duplicates all the items, except for the one at index 3, into a new array.
deleting a specific element from an array
In contrast to the earlier scenario, this code will remove the element by considering its value. However, it cannot handle duplicates as it is necessary to determine the size of the array after deletion.
package com.scdev.java;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = new int[]{1,2,3,4,5};
int[] arr_new = new int[arr.length-1];
int j=3;
for(int i=0, k=0;i<arr.length;i++){
if(arr[i]!=j){
arr_new[k]=arr[i];
k++;
}
}
System.out.println("Before deletion :" + Arrays.toString(arr));
System.out.println("After deletion :" + Arrays.toString(arr_new));
}
}
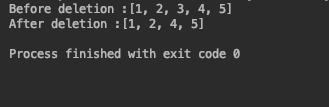
The sole distinction between this and the previous scenario is that arr[i]!=j is used in the if condition instead of i!=j.
3. When there are duplicates in the array, removing an element based on its value.
When it comes to removing duplicates based on their value, one option is to use an ArrayList. The advantage of using an ArrayList is that it doesn’t require the size to be known in advance, allowing for dynamic expansion.
package com.scdev.java;
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = new int[]{1,3,3,4,5};
ArrayList<Integer> arr_new = new ArrayList<>();
int j=3;
for(int i=0;i<arr.length;i++){
if(arr[i]!=j){
arr_new.add(arr[i]);
}
}
System.out.println("Before deletion :" + Arrays.toString(arr));
System.out.println("After deletion :" +arr_new);
}
}
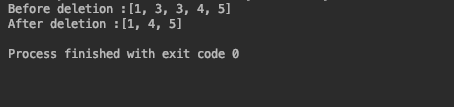
4. Changing the positions of elements within a single array.
This technique entails moving the elements within the array itself. The process of shifting elements refers to replacing the element to be removed with the element located at the next index.
package com.scdev.java;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = new int[]{1,3,3,4,5};
int j=3;
System.out.println("Before deletion :" + Arrays.toString(arr));
int count =0;
for(int i = 0; i < arr.length; i++){
if(arr[i] == j){
count++;
// shifting elements
for(int k = i; k < arr.length - 1; k++){
arr[k] = arr[k+1];
}
i--;
// break;
}
}
System.out.print("After Deletion :" );
for(int i = 0; i < arr.length-count; i++){
System.out.print(" " + arr[i]);
}
System.out.println();
System.out.println("Whole array :" + Arrays.toString(arr));
}
}
The count variable signifies the amount of elements that have been removed. It is crucial for keeping track of the index until which the array needs to be displayed. This technique also handles duplicates effectively.
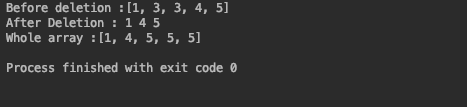
5. Removing elements from an ArrayList.
An ArrayList is supported by arrays, and removing an element from the ArrayList is a simple process that only involves making one call to an existing function.
package com.scdev.java;
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = new int[]{1,3,3,4,5};
ArrayList<Integer> arr_new = new ArrayList<Integer>();
for (int i : arr)
{
arr_new.add(i);
}
arr_new.remove(3);
System.out.println("Before deletion :" + Arrays.toString(arr));
System.out.println("After Deletion:" + arr_new);
}
}
The remove(i) function in ArrayLists eliminates the item at index i, making deletion easier in comparison to Arrays.
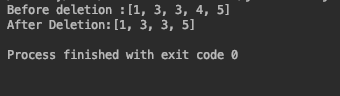
In summary, to wrap things up
We witnessed various ways to remove elements from an array. The disparity between removing an element from an Array versus an ArrayList is readily noticeable. If frequent deletions are needed, using ArrayList is advantageous as it offers built-in functions. The same applies to adding elements to an array. The dynamic nature of ArrayList allows for simpler and less burdensome ways to modify an array.
More tutorials
2D array for C++ implementation(Opens in a new browser tab)
Addition Assignment Operator mean in Java(Opens in a new browser tab)
Java Tutorial for beginners(Opens in a new browser tab)
numpy.ones() in Python(Opens in a new browser tab)
convert string to character array in Java.(Opens in a new browser tab)