How to read CSV files in Python
CSV files are frequently employed for storing tabular data in a file. Transferring data from database tables or excel files to CSV files is a straightforward process. CSV files are both human-readable and easily processed by programs. This tutorial will focus on comprehending CSV files using Python.
Can you explain what Parsing is?
To parse a file is to extract information from it. The file could include written content referred to as text files or it could be a spreadsheet.
What does the term “CSV file” refer to?
CSV, or Comma Separated Files, is a file format where data is separated by commas. This format is commonly used for handling large amounts of data. CSV files can be easily converted into spreadsheets or databases for export, or imported into other programs for use. To parse a CSV file in Python, it is relatively simple. Python has a built-in CSV library that allows for both reading from and writing to CSV files. The library provides various formats for CSV files, making data processing easy and user-friendly.
Reading a CSV file in Python
Using the built-in Python CSV module for reading CSV files.
import csv
with open('university_records.csv', 'r') as csv_file:
reader = csv.reader(csv_file)
for row in reader:
print(row)
Result:
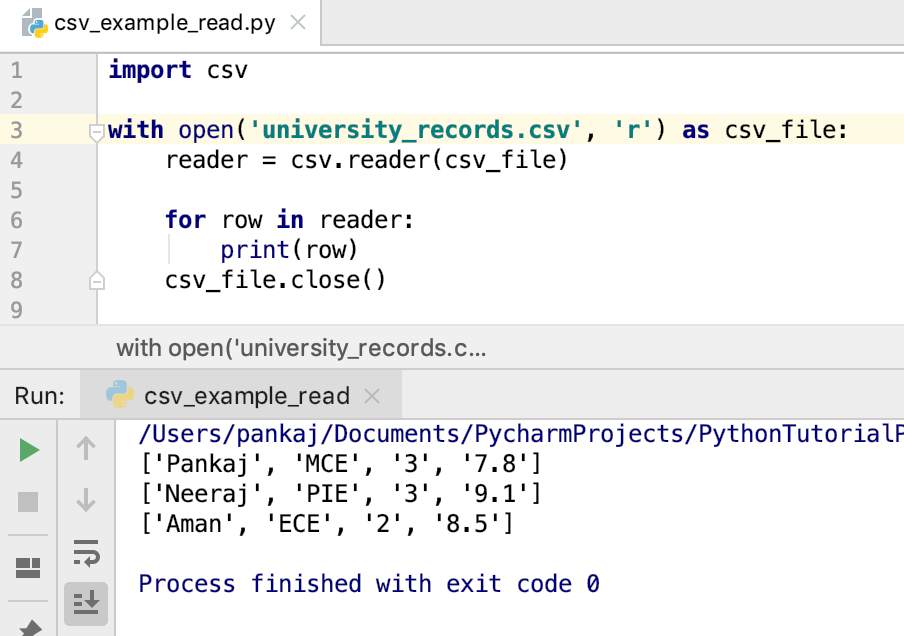
Creating a CSV file using Python
To write a file, we need to open it in either write mode or append mode. In this case, we will append the data to the current CSV file.
import csv
row = ['David', 'MCE', '3', '7.8']
row1 = ['Lisa', 'PIE', '3', '9.1']
row2 = ['Raymond', 'ECE', '2', '8.5']
with open('university_records.csv', 'a') as csv_file:
writer = csv.writer(csv_file)
writer.writerow(row)
writer.writerow(row1)
writer.writerow(row2)
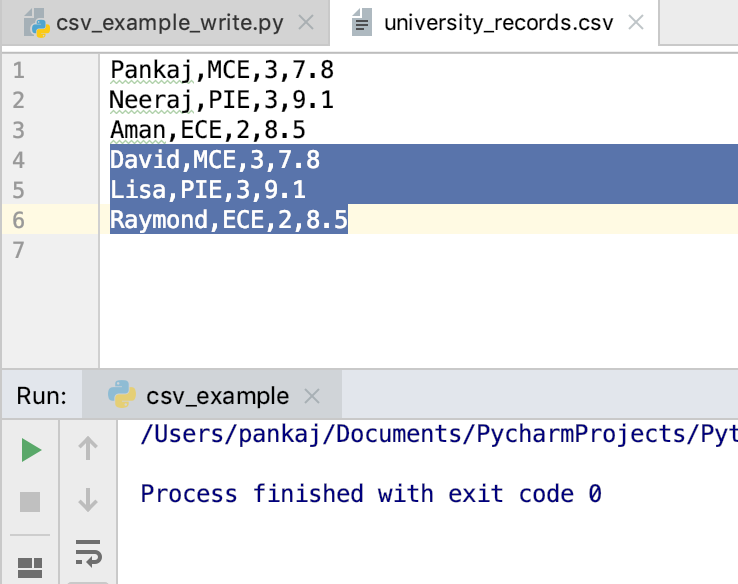
Use the Pandas library to analyze CSV files.
Using the pandas library is another way to handle CSV files, and it is widely used and considered more professional. Pandas, a Python data analysis library, provides various structures, tools, and functions for working with and modifying data, particularly tables that are either two-dimensional or one-dimensional.
The application and characteristics of the pandas Library.
- Data sets pivoting and reshaping.
- Data manipulation with indexing using DataFrame objects.
- Data filtration.
- Merge and join operation on data sets.
- Slicing, indexing, and subset of massive datasets.
- Missing data handling and data alignment.
- Row/Column insertion and deletion.
- One-Dimensional different file formats.
- Reading and writing tools for data in various file formats.
Installing pandas is a straightforward process, simply follow the instructions below to install it using PIP in order to work with the CSV file.
$ pip install pandas
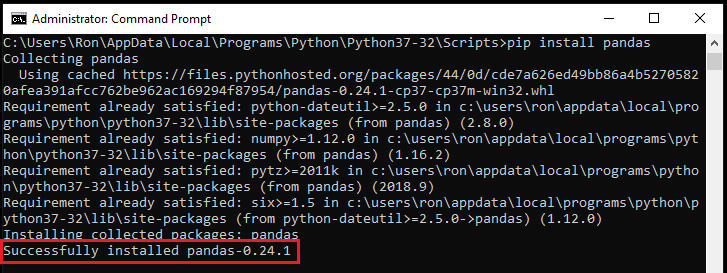
Once the installation is finished, you are ready to proceed.
Using the Pandas Module to extract data from a CSV file.
Before using pandas to import your CSV file data, it is necessary for you to have knowledge of the file’s location in your filesystem and your current working directory. It is recommended to keep your code and data file in the same directory or folder so that you can avoid specifying the path, ultimately saving time and space.
import pandas
result = pandas.read_csv('ign.csv')
print(result)
Provide a single option for rephrasing the word “Output” natively.
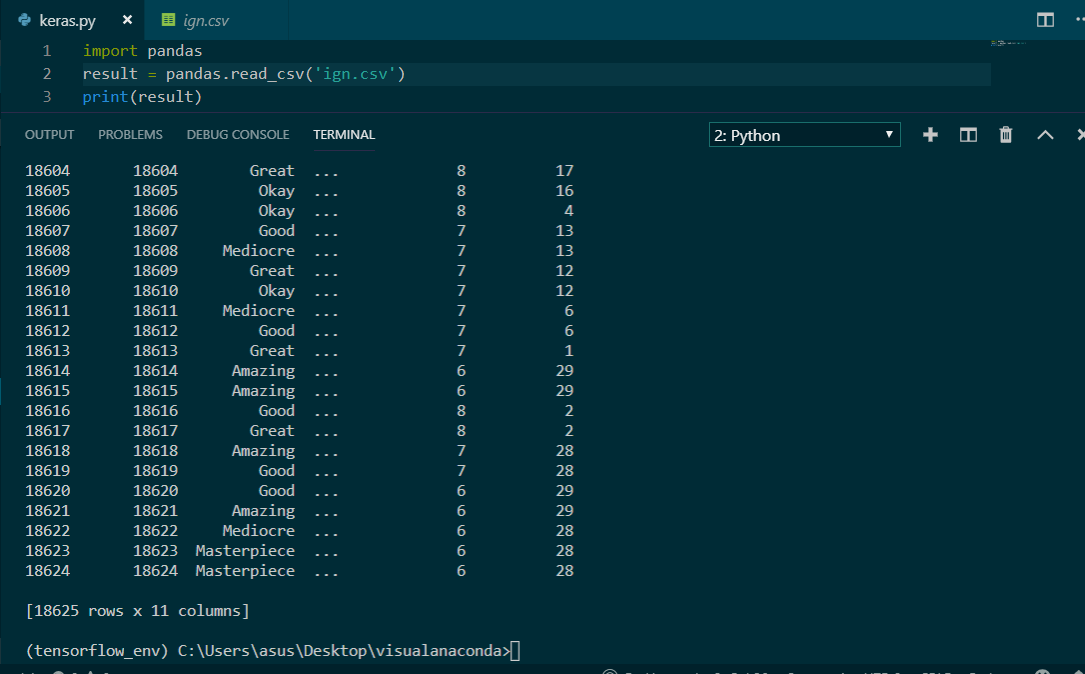
Using the Pandas Module to create a CSV file.
Using pandas, creating CSV files can be done as easily as reading them. The sole new term introduced is DataFrame. A two-dimensional, varied tabular data structure is referred to as a Pandas DataFrame (wherein data is organized in rows and columns). It comprises three principal elements: data, columns, and rows, with a labeled x-axis and y-axis (representing columns and rows).
from pandas import DataFrame
C = {'Programming language': ['Python', 'Java', 'C++'],
'Designed by': ['Guido van Rossum', 'James Gosling', 'Bjarne Stroustrup'],
'Appeared': ['1991', '1995', '1985'],
'Extension': ['.py', '.java', '.cpp'],
}
df = DataFrame(C, columns=['Programming language', 'Designed by', 'Appeared', 'Extension'])
export_csv = df.to_csv(r'program_lang.csv', index=None, header=True)
Please provide a sentence or a phrase that you would like me to paraphrase.
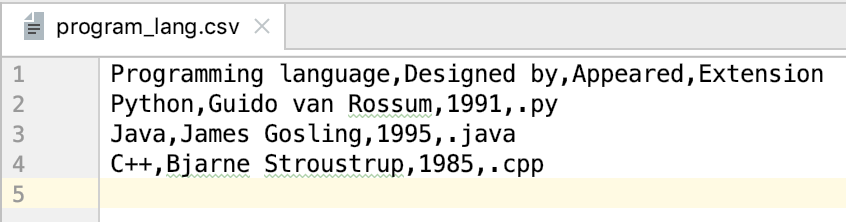
In summary
We were taught how to parse a CSV file using the built-in CSV module and pandas module. Although there are multiple ways to parse files, they are not widely used by programmers. Libraries such as PlyPlus, PLY, and ANTLR are some examples of libraries used for parsing text data. Now that you know how to use the built-in CSV library and powerful pandas module for reading and writing data in CSV format, the code provided above is basic and straightforward. It can be understood by anyone familiar with Python, so there is no need for explanation. However, manipulating complex data with empty or ambiguous entries is not easy. It requires practice and knowledge of various tools in pandas. CSV is the preferred format for saving and sharing data, and pandas is an excellent alternative to CSV modules. It may seem difficult at first, but with some practice, you will become proficient in it.
More tutorials
A tutorial on the Python Pandas module.(Opens in a new browser tab)
Reading and Writing data using Python(Opens in a new browser tab)
get pandas DataFrame from an API endpoint that lacks order?(Opens in a new browser tab)
How can you determine the standard deviation in R?(Opens in a new browser tab)
3 Simple Methods to Generate a Subset of a Python Dataframe(Opens in a new browser tab)