Joining a list in Python
When you join a list in Python, it refers to combining a series of strings with a chosen separator to create a single string. This is often handy when you need to convert a list into a string format. For instance, you can transform a list of letters into a string separated by commas and then store it in a file.
Concatenate Python List
To combine a list of strings in Python, the string join() function can be utilized. This function accepts an iterable as its argument, and since a list is an iterable, it can be used with a list. It is important to note that the list must consist of strings; attempting to join a list of integers will result in an error message: TypeError: sequence item 0: expected str instance, int found. Let’s examine a brief example demonstrating how to join a list in Python and create a string.
vowels = ["a", "e", "i", "o", "u"]
vowelsCSV = ",".join(vowels)
print("Vowels are = ", vowelsCSV)
When the program above is executed, it will generate the subsequent output.
Vowels are = a,e,i,o,u
Combine two strings in Python.
We can also make use of the join() function to combine two strings.
message = "Hello ".join("World")
print(message) #prints 'Hello World'
Why is the join() function placed in the String class rather than the List class?
Many Python developers may wonder why the join() function is included in the String class rather than the list. Wouldn’t it be simpler and more intuitive to have the following syntax to remember and use?
vowelsCSV = vowels.join(",")
I came across a well-known question on StackOverflow regarding this matter, and I have summarized the essential points from the discussions that greatly resonated with me.
The primary explanation is that the join() function can be applied to a variety of collections and will consistently produce a String outcome. Therefore, it is more logical to incorporate this function into the String API rather than duplicating it in each iterable class.
Combining a collection of diverse data types.
We will examine a program that attempts to combine elements in a list that have different types of data.
names = ['Java', 'Python', 1]
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
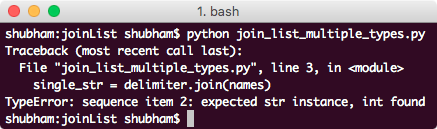
Using the join function to split a string.
The split function can also be utilized to separate a string using a specific delimiter.
names = 'Python'
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
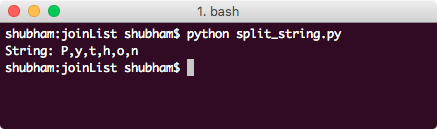
Using the split() function
In addition to using the join() function, the split() function can also be used to split a String. The split() function works in a similar manner to the join() function. Here is an example code snippet:
names = ['Java', 'Python', 'Go']
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
split = single_str.split(delimiter)
print('List: {0}'.format(split))
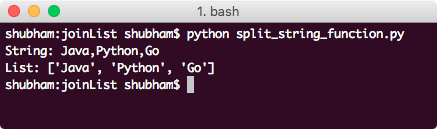
Splitting n times only.
In the previous example, we also showed how the split() function can be used with a second optional argument to indicate the number of times the split operation should be executed. Below is a sample program that showcases its usage.
names = ['Java', 'Python', 'Go']
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
split = single_str.split(delimiter, 1)
print('List: {0}'.format(split))
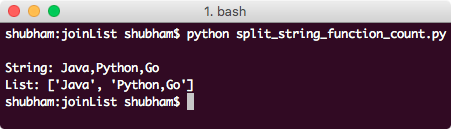
More tutorials
strsplit function in R(Opens in a new browser tab)
Adding a string to a Python variable(Opens in a new browser tab)
Java Thread Join method(Opens in a new browser tab)
Comprehending Java’s Data Types(Opens in a new browser tab)
How to include items to a list in Python(Opens in a new browser tab)