Python Keywords and Identifiers have been revised.
In this Python tutorial, we have a comprehensive guide on the installation and setup process for beginners. Now, let’s discuss Python keywords and identifiers.
Python Keywords can be restated as Reserved Words in Python.
In basic terms, Python keywords refer to words that are reserved, meaning they cannot be utilized as names for variables, classes, or functions.
You may be wondering about the purpose of these keywords. They are utilized to define the syntax and structures of the Python language.
As of the creation of this tutorial, it is important for you to be aware that Python programming language currently consists of 33 keywords. However, this number may change over time. It is crucial to note that keywords in Python are case sensitive, which means they must be written exactly as they are. Provided below is a comprehensive inventory of all the keywords in Python programming.
Don’t attempt to comprehend all the keywords in one go as it will be too much to handle. Simply acknowledge that these are the keywords for now. We will later understand how to use each of them. You can find the python keyword list by using the help function in the python shell.
All Python Keywords Compilation
and | Logical operator |
as | Alias |
assert | For debugging |
break | Break out of Python loops |
class | Used for defining Classes in Python |
continue | Keyword used to continue with the Python loop by skipping the existing |
def | Keyword used for defining a function |
del | Used for deleting objects in Python |
elif | Part of the if-elif-else conditional statement in Python |
else | Same as above |
except | A Python keyword used to catch exceptions |
FALSE | Boolean value |
finally | This keyword is used to run a code snippet when no exceptions occur |
for | Define a Python for loop |
from | Used when you need to import only a specific section of a module |
global | Specify a variable scope as global |
if | Used for defining an “if” condition |
import | Python keyword used to import modules |
in | Checks if specified values are present in an iterable object |
is | This keyword is used to test for equality. |
lambda | Create anonymous functions |
None | The None keyword represents a Null value in PYthon |
nonlocal | Declare a variable with non-local scope |
not | Logical operator to negate a condition |
or | A logical operator used when either one of the conditions needs to be true |
pass | This Python keyword passes and lets the function continue further |
raise | Raises an exception when called with the specified value |
return | Exits a running function and returns the value specified |
TRUE | Boolean value |
try | Part of the try…except statement |
while | Used for defining a Python while loop |
with | Creates a block to make exception handling and file operations easy |
yield | Ends a function and returns a generator object |
Here is a basic illustration demonstrating the implementation of if-else in a python program.
var = 1;
if(var==1):
print("odd")
else:
print("even")
Once the program is executed, Python is able to comprehend the if-else block due to its predefined words and structure, and proceeds with any additional operations.
What do Python Identifiers refer to?
We refer to Python Identifier as the designation used to identify a variable, function, class, module, or any other object. In simple terms, whenever we wish to assign a name to an entity, it is known as an identifier.
Variable and identifier are frequently mistaken for being the same, but they are actually distinct. To clarify, let’s examine the definition of a variable.
What does the term “Variable” mean in Python?
In its essence, a variable is something that can be altered as time progresses. Specifically, it acts as a designated spot in memory where a value can be saved. Later on, we can access this value for utilization. However, to accomplish this, we must assign a unique name to this memory location, which is referred to as an identifier.
Guidelines for Writing Identifiers
Before discussing the rules for writing identifiers in Python, it is important to understand that Python is case-sensitive. This implies that “Name” and “name” are considered as two distinct identifiers in Python. Now, let’s proceed with the rules for writing Python identifiers.
- Python identifiers can consist of a mix of uppercase and lowercase letters, digits, or an underscore (_). Hence, examples such as myVariable, variable_1, and variable_for_print are considered valid identifiers. However, an identifier cannot begin with a digit, so while variable1 is acceptable, 1variable is not. Additionally, special symbols like !, #, @, %, and $ cannot be used in identifiers. Identifiers can have varying lengths.
While these rules for writing identifiers may be stringent, there are also optional naming conventions that are recommended to be followed.
-
- Class names begin with a capital letter, while all other identifiers begin with a lowercase letter.
-
- An identifier prefixed with a single underscore implies that it is private.
-
- If an identifier is surrounded by double underscores, it is a special name defined by the language.
-
- Although c = 10 is acceptable, using a more meaningful name like count = 10 would be clearer and easier to understand, especially when revisiting the code after a while.
- To separate multiple words in an identifier, an underscore can be used, for instance this_is_a_variable.
Here’s an example code for Python variables.
myVariable="hello world"
print(myVariable)
var1=1
print(var1)
var2=2
print(var2)
If you execute the program, the output will resemble the image provided.
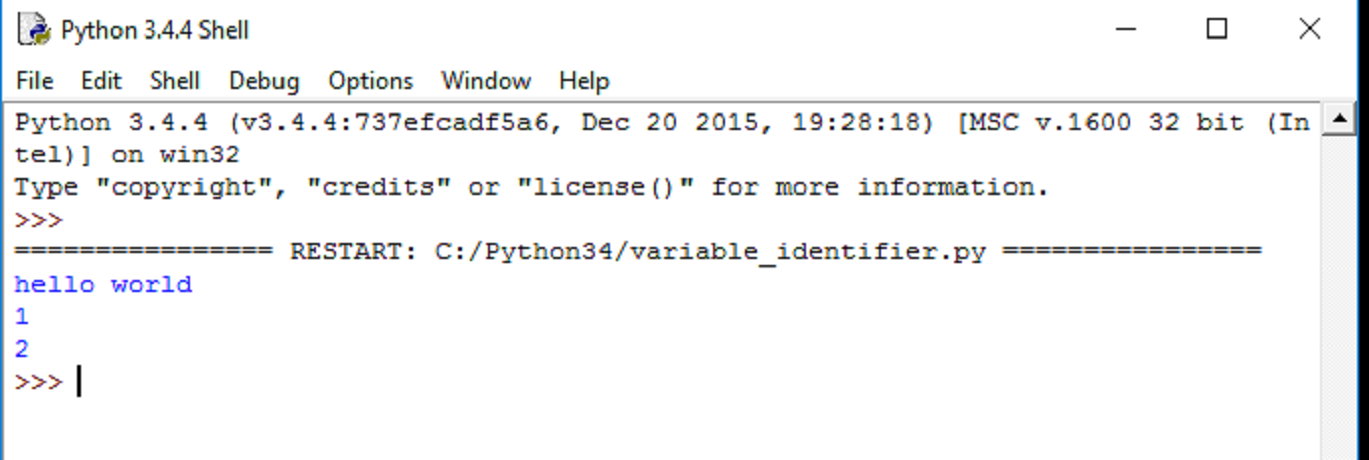
Summary
Result
That concludes today’s lesson. In the next tutorial, we will explore Python Statements and Comments. Until then, happy coding!
more tutorials
Frequently Asked Questions about Writing for Donations(Opens in a new browser tab)
Python HTTP requests such as GET and POST methods.(Opens in a new browser tab)
Set in Python(Opens in a new browser tab)
Converting a Python string to an integer and vice versa.(Opens in a new browser tab)
How to include items to a list in Python(Opens in a new browser tab)