Reading and Writing data using Python
In this tutorial, we will focus on various file operations within Python. We will cover reading, writing, and deleting files using Python, along with other functionalities. So, without wasting any time, let us begin.
Python file manipulation
In the tutorial before, we utilized the console for input. However, in this lesson, we will obtain input from a file. Consequently, we will be able to read and write data to files. To accomplish this, we must adhere to a set of instructions. These instructions include:
-
- 1. Begin by accessing a file
-
- Receive input from the file / Produce output to the file
- Conclude by closing the file
We will also cover important actions like file copying and file deletion during our learning process.
What is the necessity for file operations in Python?
To effectively handle extensive datasets in machine learning tasks, it is essential to manipulate files. Given that Python is a widely utilized language in the field of data science, it is crucial to possess proficiency in the various file operations provided by Python.
Therefore, we will now delve into various Python file operations.
Use the open() function in Python to access and read a file.
To begin utilizing files in Python, it is essential to master the skill of file opening. The open() method enables file opening.
In Python, the open() function has two parameters to pass. The initial parameter is the full path of the file, including its name, and the second parameter is the mode in which the file is opened.
I have outlined below some of the typical ways of reading files.
- ‘r’ : This mode indicate that file will be open for reading only
- ‘w’ : This mode indicate that file will be open for writing only. If file containing containing that name does not exists, it will create a new one
- ‘a’ : This mode indicate that the output of that program will be append to the previous output of that file
- ‘r+’ : This mode indicate that file will be open for both reading and writing
Moreover, in the Windows OS, you may add ‘b’ to access the file in binary format. This distinction is necessary as Windows distinguishes between binary text files and standard text files.
Imagine that we have a text file named ‘file.txt’ in the exact folder where our code is located. Our intention is to access and open that particular file.
The function open(filename, mode) returns a file object which allows you to perform additional operations.
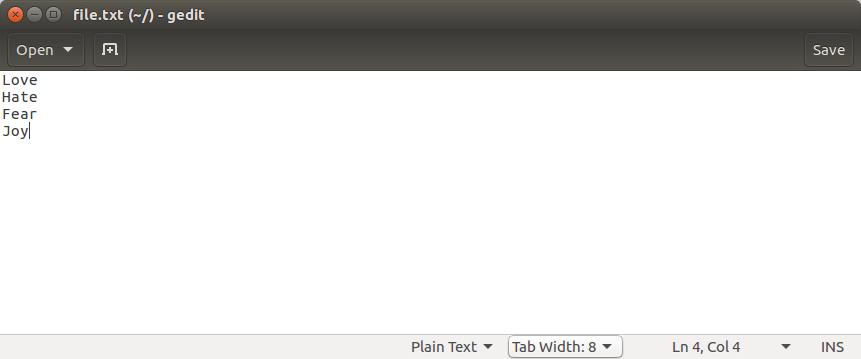
#directory: /home/imtiaz/code.py
text_file = open('file.txt','r')
#Another method using full location
text_file2 = open('/home/imtiaz/file.txt','r')
print('First Method')
print(text_file)
print('Second Method')
print(text_file2)
The result of the code will be.
================== RESTART: /home/imtiaz/code.py ==================
First Method
Second Method
>>>
2. In Python, you can both read from and write to files.
Python provides different functions for reading and writing files, and each function performs distinct actions. It is crucial to consider the file operation mode. If you want to read a file, you must open it in either read or write mode. On the other hand, when writing to a file in Python, it is necessary for the file to be open in write mode.
Below are several Python functions that enable file reading and writing:
- read() : This function reads the entire file and returns a string
- readline() : This function reads lines from that file and returns as a string. It fetch the line n, if it is been called nth time.
- readlines() : This function returns a list where each element is single line of that file.
- readlines() : This function returns a list where each element is single line of that file.
- write() : This function writes a fixed sequence of characters to a file.
- writelines() : This function writes a list of string.
- append() : This function append string to the file instead of overwriting the file.
For instance, we can consider a file called “abc.txt” and utilize a for loop to read each line of the file.
#open the file
text_file = open('/Users/scdev/abc.txt','r')
#get the list of line
line_list = text_file.readlines();
#for each line from the list, print the line
for line in line_list:
print(line)
text_file.close() #don't forget to close the file
What is produced:
Now that we have learned how to read a file in Python, let us proceed and execute a write operation utilizing the writelines() function.
#open the file
text_file = open('/Users/scdev/file.txt','w')
#initialize an empty list
word_list= []
#iterate 4 times
for i in range (1, 5):
print("Please enter data: ")
line = input() #take input
word_list.append(line) #append to the list
text_file.writelines(word_list) #write 4 words to the file
text_file.close() #don’t forget to close the file
One possible paraphrase of “Output” could be “Result.”
3. Use the shutil() method in Python to replicate files.
The shutil module in Python enables us to copy files. It provides us with the ability to perform copy and move actions on various files. To illustrate, let’s proceed with an example.
import shutil
shutil.copy2('/Users/scdev/abc.txt', '/Users/scdev/abc_copy2.txt')
#another way to copy file
shutil.copyfile('/Users/scdev/abc.txt', '/Users/scdev/abc_copyfile.txt')
print("File Copy Done")
4. You can use the shutil.os.remove() method in Python to eliminate files.
The remove() method from Python’s shutil module provides the capability to delete files from the file system. Now, we will explore how we can carry out a deletion task in Python.
import shutil
import os
#two ways to delete file
shutil.os.remove('/Users/scdev/abc_copy2.txt')
os.remove('/Users/scdev/abc_copy2.txt')
5. Use the close() method in Python to end an open file.
In Python, it is crucial to close a file after making modifications. By doing so, you retain the changes, remove the file from memory, and prevent any further reading or writing within the program.
To end an open file in Python, utilize the syntax:
fileobject.close()
To build upon our prior instances of file reading, here is one way to conclude the file:
text_file = open('/Users/scdev/abc.txt','r')
# some file operations here
text_file.close()
Moreover, if you utilize the with block, there is no need for manual file closure. Once the with block is executed, the files will be automatically closed and unable to be accessed for reading or writing.
The Python program encounters a FileNotFoundError.
Receiving the FileNotFoundError is a usual occurrence when dealing with files in Python. However, you can easily prevent this by ensuring you provide complete file paths while creating the file object.
File "/Users/scdev/Desktop/string1.py", line 2, in <module>
text_file = open('/Users/scdev/Desktop/abc.txt','r')
FileNotFoundError: [Errno 2] No such file or directory: '/Users/scdev/Desktop/abc.txt'
To resolve the FileNotFoundError, all you have to do is ensure that the path specified in the file open method is accurate.
In conclusion
Here are the available file operations in Python. Python offers numerous other ways to work with files, such as reading CSV data and more. This article explains how you can utilize the Pandas module to read CSV datasets in Python.
I hope you had a good time reading the article! Enjoy your learning journey 🙂
One possible paraphrase could be:
– You can find more information about reading and writing files in Python at this link: https://docs.python.org/3/tutorial/inputoutput.html#reading-and-writing-files.
More Tutorials
How can properties files be read in Python?(Opens in a new browser tab)
How to enable a port on a Linux operating system.(Opens in a new browser tab)
Frequently Asked Questions about Writing for Donations(Opens in a new browser tab)
Set in Python(Opens in a new browser tab)
How can I utilize PhotoRec to retrieve erased files?(Opens in a new browser tab)