Square Root of Matrix Elements with NumPy sqrt() function.
The Python NumPy module serves the purpose of working with arrays of multiple dimensions and manipulating matrices. By employing the NumPy sqrt() function, we are able to obtain the square root of the elements within the matrix.
Example of using the sqrt() function in Python‘s NumPy.
import numpy
array_2d = numpy.array([[1, 4], [9, 16]], dtype=numpy.float)
print(array_2d)
array_2d_sqrt = numpy.sqrt(array_2d)
print(array_2d_sqrt)
One possibility for paraphrasing the given statement natively is:
Result:
[[ 1. 4.]
[ 9. 16.]]
[[1. 2.]
[3. 4.]]
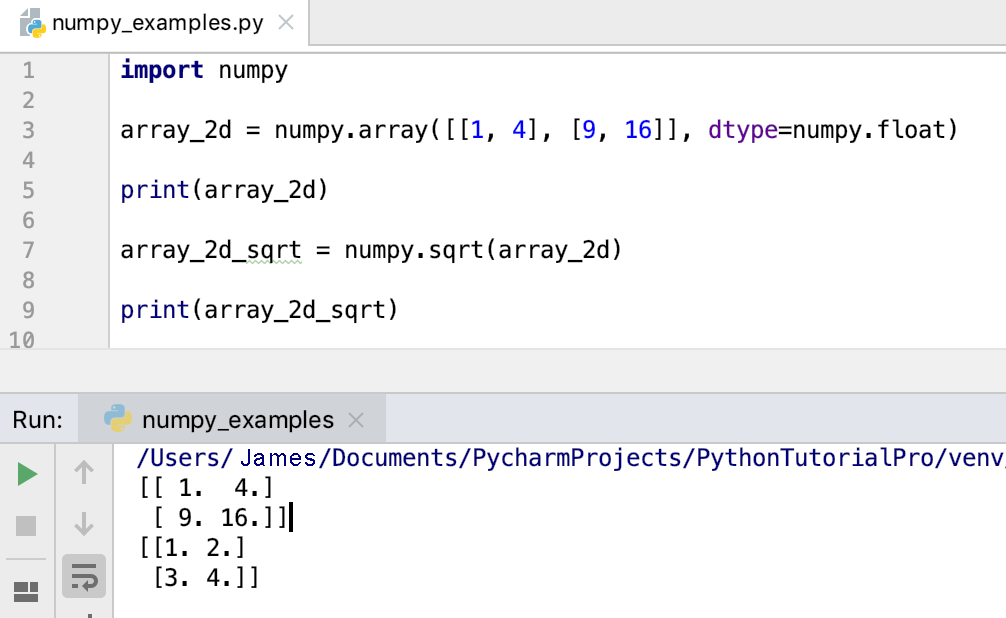
Now, let’s examine another instance where the elements of the matrix are not the squares of integers. In this case, we will utilize the Python interpreter.
>>> import numpy
>>>
>>> array = numpy.array([[1, 3], [5, 7]], dtype=numpy.float)
>>>
>>> print(array)
[[1. 3.]
[5. 7.]]
>>>
>>> array_sqrt = numpy.sqrt(array)
>>>
>>> print(array_sqrt)
[[1. 1.73205081]
[2.23606798 2.64575131]]
>>>
One option for paraphrasing the phrase “NumPy sqrt() Infinity Example” natively could be:
“An example demonstrating the use of the square root function (sqrt) in NumPy with the concept of infinity.”
Let’s observe the outcome when we consider infinity as the matrix element.
>>> array = numpy.array([1, numpy.inf])
>>>
>>> numpy.sqrt(array)
array([ 1., inf])
>>>
Complex numbers can be expressed in a more elaborate manner.
>>> array = numpy.array([1 + 2j, -3 + 4j], dtype=numpy.complex)
>>>
>>> numpy.sqrt(array)
array([1.27201965+0.78615138j, 1. +2.j ])
>>>

Numbers less than zero.
>>> array = numpy.array([4, -4])
>>>
>>> numpy.sqrt(array)
__main__:1: RuntimeWarning: invalid value encountered in sqrt
array([ 2., nan])
>>>
If a matrix contains negative numbers, taking its square root will result in a RuntimeWarning, and the square root of each element will be returned as “nan” (not a number) according to the NumPy documentation.
More tutorials
ValueError exception in Python code examples(Opens in a new browser tab)
numpy.ones() in Python(Opens in a new browser tab)
2D array for C++ implementation(Opens in a new browser tab)
A tutorial on the Python Pandas module.(Opens in a new browser tab)
How to include items to a list in Python(Opens in a new browser tab)