JavaのListIteratorは、JavaのListIteratorです。
私たちはJavaには4種類のカーソルがあることを知っています。Enumeration、Iterator、ListIterator、およびSpliteratorです。私は以前の投稿でEnumerationとIteratorのカーソルについてすでに説明しましたので、この投稿を読む前に、Java Iteratorで私の前の投稿をお読みください。この投稿では、3番目のJavaカーソルであるListIteratorについて議論します。
Java の ListIterator
リストイテレータはJavaのイテレータであり、リスト実装オブジェクトから要素を1つずつ反復処理するために使用されます。
- It is available since Java 1.2.
- It extends Iterator interface.
- It is useful only for List implemented classes.
- Unlike Iterator, It supports all four operations: CRUD (CREATE, READ, UPDATE and DELETE).
- Unlike Iterator, It supports both Forward Direction and Backward Direction iterations.
- It is a Bi-directional Iterator.
- It has no current element; its cursor position always lies between the element that would be returned by a call to previous() and the element that would be returned by a call to next().
コレクションAPIにおけるCRUD操作とは何ですか?
- CREATE: Adding new elements to Collection object.
- READ: Retrieving elements from Collection object.
- UPDATE: Updating or setting existing elements in Collection object.
- DELETE: Removing elements from Collection object.
JavaのListIteratorクラスのダイアグラム
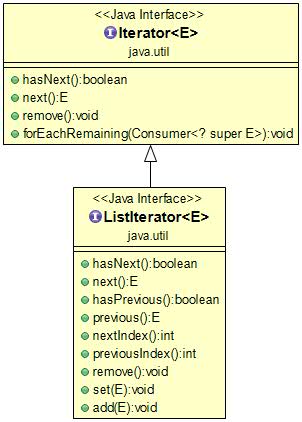
JavaのListIteratorメソッド
JavaのListIteratorインターフェースには、以下のメソッドがあります。
- void add(E e): Inserts the specified element into the list.
- boolean hasNext(): Returns true if this list iterator has more elements when traversing the list in the forward direction.
- boolean hasPrevious(): Returns true if this list iterator has more elements when traversing the list in the reverse direction.
- E next(): Returns the next element in the list and advances the cursor position.
- int nextIndex(): Returns the index of the element that would be returned by a subsequent call to next().
- E previous(): Returns the previous element in the list and moves the cursor position backwards.
- int previousIndex(): Returns the index of the element that would be returned by a subsequent call to previous().
- void remove(): Removes from the list the last element that was returned by next() or previous().
- void set(E e): Replaces the last element returned by next() or previous() with the specified element.
次のセクションでは、役立つ例を交えながら、これらの方法を一つずつ探っていきます。
JavaのListIteratorの基本的な例
このセクションでは、いくつかのListIteratorのメソッドについていくつかの例を紹介します。まずは、このイテレーターオブジェクトを取得する方法について理解する必要があります。ListIteratorをどのように取得するのか。
ListIterator<E> listIterator()
このリストの要素に対するリストイテレータを返します。例:
import java.util.*;
public class ListIteratorDemo
{
public static void main(String[] args)
{
List<String> names = new LinkedList<>();
names.add("Rams");
names.add("Posa");
names.add("Chinni");
// Getting ListIterator
ListIterator<String> namesIterator = names.listIterator();
// Traversing elements
while(namesIterator.hasNext()){
System.out.println(namesIterator.next());
}
// Enhanced for loop creates Internal Iterator here.
for(String name: names){
System.out.println(name);
}
}
}
出力:
Rams
Posa
Chinni
リストのイテレーター双方向イテレーションの例
このセクションでは、ListIteratorのメソッドが前方向と後方向の反復を行う方法を探求します。
import java.util.*;
public class BiDirectinalListIteratorDemo
{
public static void main(String[] args)
{
List<String> names = new LinkedListt<>();
names.add("Rams");
names.add("Posa");
names.add("Chinni");
// Getting ListIterator
ListIterator<String> listIterator = names.listIterator();
// Traversing elements
System.out.println("Forward Direction Iteration:");
while(listIterator.hasNext()){
System.out.println(listIterator.next());
}
// Traversing elements, the iterator is at the end at this point
System.out.println("Backward Direction Iteration:");
while(listIterator.hasPrevious()){
System.out.println(listIterator.previous());
}
}
}
出力:
Forward Direction Iteration:
Rams
Posa
Chinni
Backward Direction Iteration:
Chinni
Posa
Rams
Java イテレータの種類
私たちが知っているように、Javaには4つのカーソルがあります。それらはEnumeration、Iterator、ListIterator、およびSpliteratorです。以下に示すように、それらを2つの主なタイプに分類することができます。
- Uni-Directional Iterators
They are Cursors which supports only Forward Direction iterations. For instance, Enumeration, Iterator, etc. are Uni-Directional Iterators.- Bi-Directional Iterators
They are Cursors which supports Both Forward Direction and Backward Direction iterations. For instance, ListIterator is Bi-Directional Iterator.
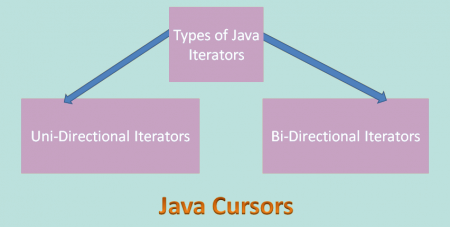
JavaのListIteratorは内部的にどのように機能しますか?
私たちが知っているように、JavaのListIteratorは正方向と逆方向の両方で動作することを意味します。これは双方向イテレータです。この機能をサポートするために、2つのメソッドセットがあります。
- Forward Direction Iteration methods
We need to use the following methods to support Forward Direction Iteration:
-
- hasNext()
-
- 次()
- 次のインデックス()
- Backward Direction Iteration methods
We need to use the following methods to support Backward Direction Iteration:
-
- 前の要素があるか確認する(hasPrevious())
-
- 前の要素を取得する(previous())
- 前の要素のインデックスを取得する(previousIndex())
以前の投稿では、「JavaのIteratorは内部的にどのように動作するか?」のセクションで、Iteratorが「前方方向に移動する方法についてすでに議論してきました。 ListIteratorも同じ方法で動作します。前の投稿を読みたい場合は、こちらをクリックしてください: JavaのIterator。このセクションでは、ListIteratorが後方方向でどのように動作するかについて議論します。この機能を理解するために、次のLinkedListオブジェクトを取り上げましょう。
List<String> names = new LinkedList<>();
names.add("E-1");
names.add("E-2");
names.add("E-3");
.
.
.
names.add("E-n");
次のように、LinkedList上でListIteratorオブジェクトを作成してください。
ListIterator<String> namesIterator = names.listLterator();
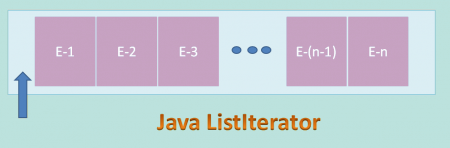
namesIterator.hasNext();
namesIterator.next();
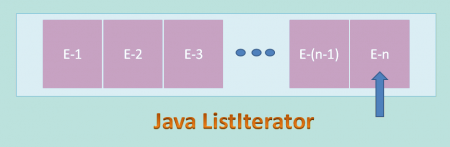
namesIterator.hasPrevious();
namesIterator.previous();
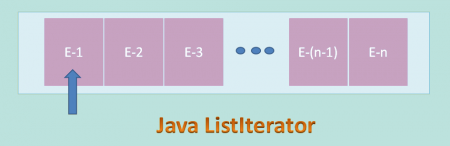
namesIterator.hasPrevious();
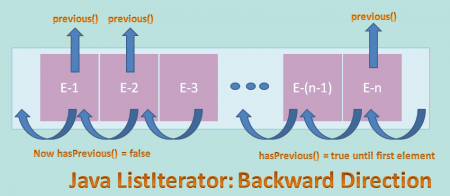
ListIteratorの利点
イテレーターとは異なり、リストイテレーターには以下の利点があります。
- Like Iterator, it supports READ and DELETE operations.
- It supports CREATE and UPDATE operations too.
- That means, it supports CRUD operations: CREATE, READ, UPDATE and DELETE operations.
- It supports both Forward direction and Backward direction iteration. That means it’s a Bi-Directional Java Cursor.
- Method names are simple and easy to use them.
ListIteratorの制約
Iteratorに比べて、JavaのListIteratorは多くの利点を持っています。ただし、以下にいくつかの制限があります。
- It is an Iterator only List implementation classes.
- Unlike Iterator, it is not applicable for whole Collection API.
- It is not a Universal Java Cursor.
- Compare to Spliterator, it does NOT support Parallel iteration of elements.
- Compare to Spliterator, it does NOT support better performance to iterate large volume of data.
IteratorとListIteratorの類似点
このセクションでは、Javaの2つのカーソル、IteratorとListIteratorの類似点について話し合います。
- Bother are introduced in Java 1.2.
- Both are Iterators used to iterate Collection or List elements.
- Both supports READ and DELETE operations.
- Both supports Forward Direction iteration.
- Both are not Legacy interfaces.
イテレータとリストイテレータの違い
このセクションでは、Javaの2つのイテレーター、IteratorとListIteratorの違いについて話し合います。
Iterator | ListIterator |
---|---|
Introduced in Java 1.2. | Introduced in Java 1.2. |
It is an Iterator for whole Collection API. | It is an Iterator for only List implemented classes. |
It is an Universal Iterator. | It is NOT an Universal Iterator. |
It supports only Forward Direction Iteration. | It supports both Forward and Backward Direction iterations. |
It’s a Uni-Directional Iterator. | It’s a Bi-Directional Iterator. |
It supports only READ and DELETE operations. | It supports all CRUD operations. |
We can get Iterator by using iterator() method. | We can ListIterator object using listIterator() method. |
これがすべてのJavaのListIteratorに関する情報です。これらのJava ListIteratorの理論や例がListIteratorプログラミングの初めに役立つことを願っています。参照:ListIterator APIドキュメント。