Javaにおけるピラミッドパターンのプログラム
パターンプログラムは、面接での面接者の論理的思考能力を理解するために非常によく使われます。ピラミッドパターンは非常に人気があり、作成方法のロジックを理解すれば、同じ結果を得るためのコードの作成は簡単な仕事です。
Javaにおけるピラミッドパターンのプログラム
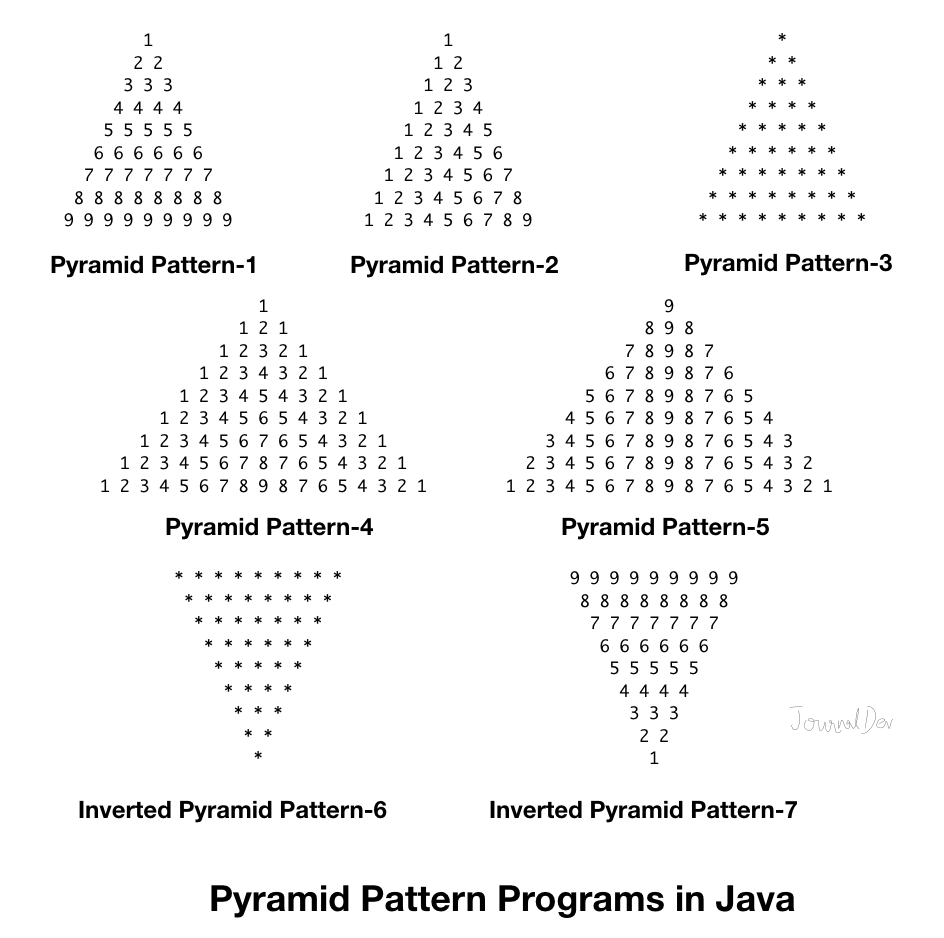
数のピラミッドパターン
最初のパターンを見ると、各行には同じ数が同じ回数印刷されています。しかし、各行には “rows-i” 回だけの前に空白スペースがあります。このパターンを出力するプログラムを見てみましょう。
package com.scdev.patterns.pyramid;
import java.util.Scanner;
public class PyramidPattern {
private static void printPattern1(int rows) {
// for loop for the rows
for (int i = 1; i <= rows; i++) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = rows - i;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print numbers
printString(i + " ", i);
//move to next line
System.out.println("");
}
}
//utility function to print string given times
private static void printString(String s, int times) {
for (int j = 0; j < times; j++) {
System.out.print(s);
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter the rows to print:");
int rows = scanner.nextInt();
// System.out.println("Rows = "+rows);
scanner.close();
System.out.println("Printing Pattern 1\n");
printPattern1(rows);
}
}
以下のように、共通の文字列出力タスクのためのユーティリティ関数を作成したことに注意してください。プログラムを短い再利用可能な関数に分割できる場合、それはプログラムを書くだけでなく、品質と再利用性にも注意を払っていることを示しています。上記のプログラムを実行すると、以下の出力が得られます。
Please enter the rows to print:
9
Printing Pattern 1
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
6 6 6 6 6 6
7 7 7 7 7 7 7
8 8 8 8 8 8 8 8
9 9 9 9 9 9 9 9 9
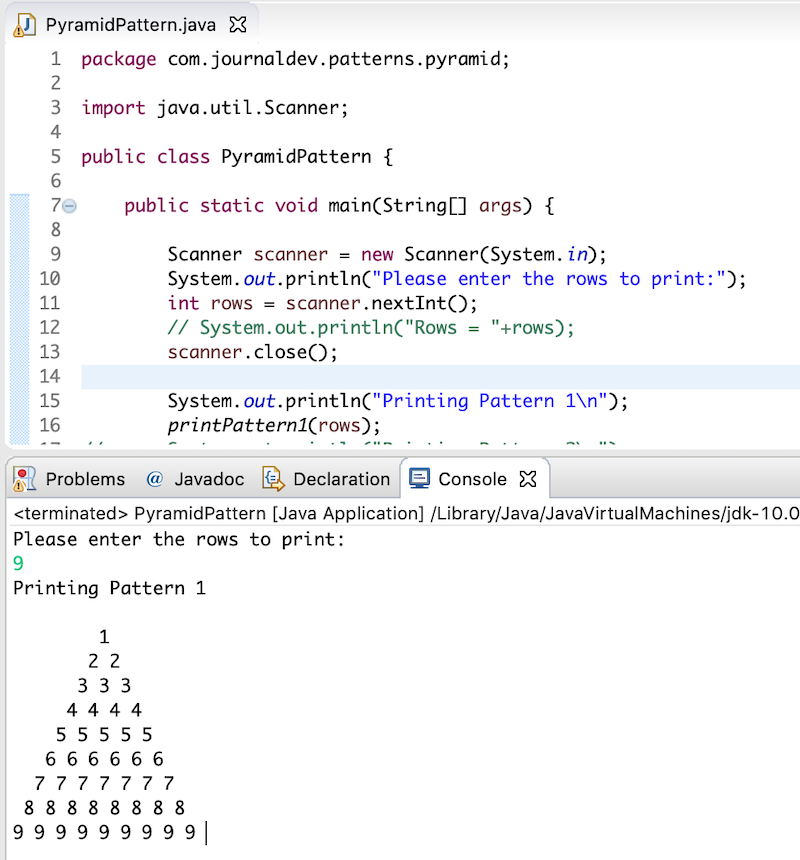
数字が増加するピラミッドパターン
こちらはパターン2を出力する関数です。注意すべきポイントは、先頭の空白の数と、その後数値が昇順に表示されることです。
/**
*
* Program to print below pyramid structure
* 1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
1 2 3 4 5 6 7
1 2 3 4 5 6 7 8
1 2 3 4 5 6 7 8 9
*/
private static void printPattern2(int rows) {
// for loop for the rows
for (int i = 1; i <= rows; i++) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = rows - i;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print numbers
for(int x = 1; x<=i; x++) {
System.out.print(x+" ");
}
//move to next line
System.out.println("");
}
}
キャラクターのピラミッド
これはとても簡単なもので、計算や操作はせずに文字だけを印刷する必要があります。
/**
* Program to print following pyramid structure
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
*/
private static void printPattern3(int rows) {
// for loop for the rows
for (int i = 1; i <= rows; i++) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = rows - i;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print character
printString("* ", i);
//move to next line
System.out.println("");
}
}
ピラミッドのパターンを作成するプログラム4
/**
*
* 1
1 2 1
1 2 3 2 1
1 2 3 4 3 2 1
1 2 3 4 5 4 3 2 1
1 2 3 4 5 6 5 4 3 2 1
1 2 3 4 5 6 7 6 5 4 3 2 1
1 2 3 4 5 6 7 8 7 6 5 4 3 2 1
1 2 3 4 5 6 7 8 9 8 7 6 5 4 3 2 1
*/
private static void printPattern4(int rows) {
// for loop for the rows
for (int i = 1; i <= rows; i++) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = (rows-i)*2;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print numbers
for(int x=1; x0; j--) {
System.out.print(j+" ");
}
//move to next line
System.out.println("");
}
}
各行には2 * r-1個の数字があることに注意してください。したがって、数字を印刷する前に(行 – i)* 2のスペースを持っています。その後、数字は1から「i」まで、そして再び1になります。これを実現するために、数字を印刷するためのロジックには2つのforループが必要です。
Javaでのピラミッドパターン5プログラム
/**
*
* 9
8 9 8
7 8 9 8 7
6 7 8 9 8 7 6
5 6 7 8 9 8 7 6 5
4 5 6 7 8 9 8 7 6 5 4
3 4 5 6 7 8 9 8 7 6 5 4 3
2 3 4 5 6 7 8 9 8 7 6 5 4 3 2
1 2 3 4 5 6 7 8 9 8 7 6 5 4 3 2 1
*/
private static void printPattern5(int rows) {
// for loop for the rows
for (int i = rows; i >= 1; i--) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = i*2;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print numbers
for(int x=i; x=i; j--) {
System.out.print(j+" ");
}
//move to next line
System.out.println("");
}
}
文字の逆ピラミッドパターン
ここに逆ピラミッドプログラムのコードスニペットがあります。
/**
*
* * * * * * * * *
* * * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
*/
private static void printPattern6(int rows) {
// for loop for the rows
for (int i = rows; i >= 1; i--) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = rows - i;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print character
printString("* ", i);
//move to next line
System.out.println("");
}
}
数字の逆ピラミッドパターン
数字で作られた逆ピラミッドパターンの例を見てみましょう。
/**
*
9 9 9 9 9 9 9 9 9
8 8 8 8 8 8 8 8
7 7 7 7 7 7 7
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
*/
private static void printPattern7(int rows) {
// for loop for the rows
for (int i = rows; i >= 1; i--) {
// white spaces in the front of the numbers
int numberOfWhiteSpaces = rows - i;
//print leading white spaces
printString(" ", numberOfWhiteSpaces);
//print character
printString(i+" ", i);
//move to next line
System.out.println("");
}
}
結論
多くの種類のピラミッドのパターンがあります。一番重要なポイントは、数字や空白がどのように配置されているかのパターンを理解することです。パターンが分かれば、Javaや他のプログラミング言語でも簡単にコードを書くことができます。もし特定のパターンのプログラムをお探しでしたら、教えていただければお手伝いいたします。
さらに読むと
Javaでの文字列プログラムとJavaプログラミングの面接質問
私たちの GitHub リポジトリから、完全なコードとさらにプログラミングの例をチェックすることができます。