Pythonのreturn文
Pythonのreturn文は、関数から値を返すために使用されます。return文は、関数の内部でのみ使用できます。Pythonの関数以外では使用できません。
返り値を持たないPythonの関数
Pythonのすべての関数は何かしらの値を返します。もし関数にreturn文がない場合、Noneが返されます。
def print_something(s):
print('Printing::', s)
output = print_something('Hi')
print(f'A function without return statement returns {output}')
出力:
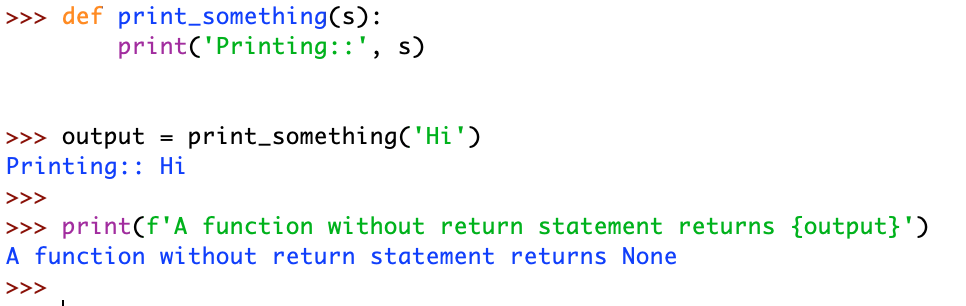
PythonのReturn文の例を示します。
return文を使用して、関数内でいくつかの操作を実行し、その結果を呼び出し元に返すことができます。
def add(x, y):
result = x + y
return result
output = add(5, 4)
print(f'Output of add(5, 4) function is {output}')
出力:
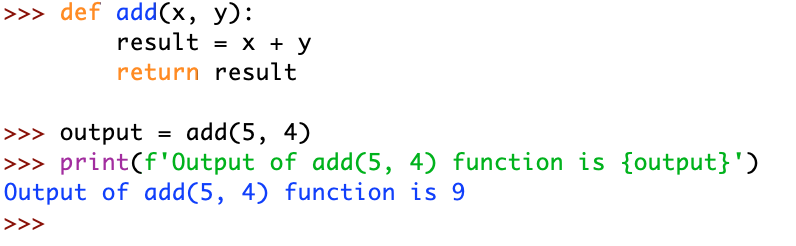
Pythonのreturn文は、式を返すことができます。
返り値の中にも式を使用することができます。その場合、式が評価されて結果が返されます。
def add(x, y):
return x + y
output = add(5, 4)
print(f'Output of add(5, 4) function is {output}')
出力:
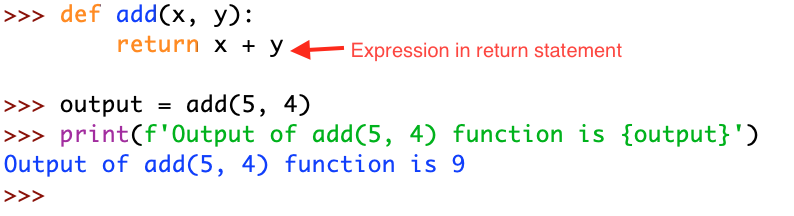
Pythonは真偽値を返す。
関数の引数のブール値を返す例を見てみましょう。オブジェクトのブール値を取得するために、bool()関数を使用します。
def bool_value(x):
return bool(x)
print(f'Boolean value returned by bool_value(False) is {bool_value(False)}')
print(f'Boolean value returned by bool_value(True) is {bool_value(True)}')
print(f'Boolean value returned by bool_value("Python") is {bool_value("Python")}')
出力:
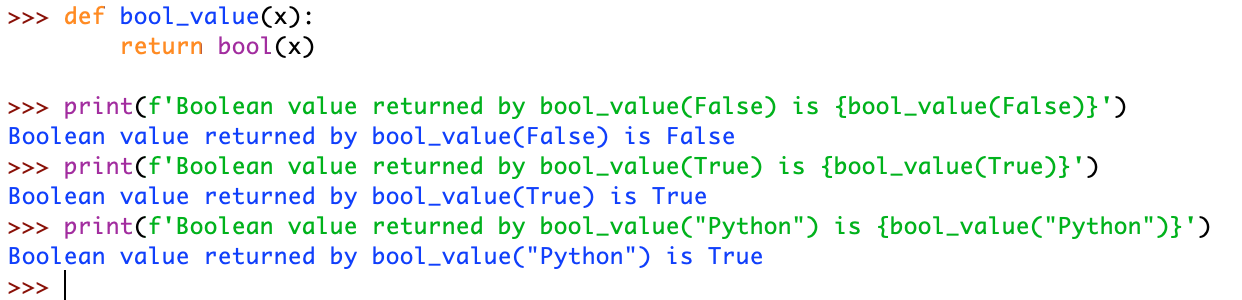
Pythonは文字列を返す
実際の例を見てみましょう。関数が引数の文字列表現を返す場合を考えてみます。str()関数を使用してオブジェクトの文字列表現を取得することができます。
def str_value(s):
return str(s)
print(f'String value returned by str_value(False) is {str_value(False)}')
print(f'String value returned by str_value(True) is {str_value(True)}')
print(f'String value returned by str_value(10) is {str_value(10)}')
出力:
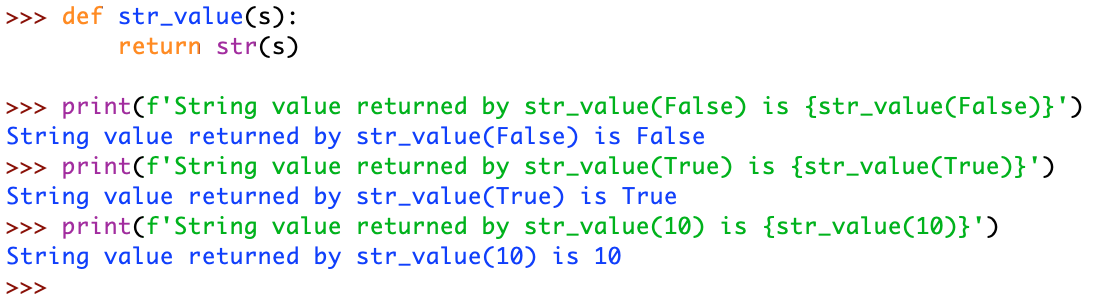
Pythonの戻り値はタプルです。
数個の変数をタプルに変換したいことがあります。可変数の引数からタプルを返す関数の書き方を見てみましょう。
def create_tuple(*args):
my_list = []
for arg in args:
my_list.append(arg * 10)
return tuple(my_list)
t = create_tuple(1, 2, 3)
print(f'Tuple returned by create_tuple(1,2,3) is {t}')
出力:
以下の文を日本語で自然に言い換えてください。一つのオプションのみ必要です。
出力:
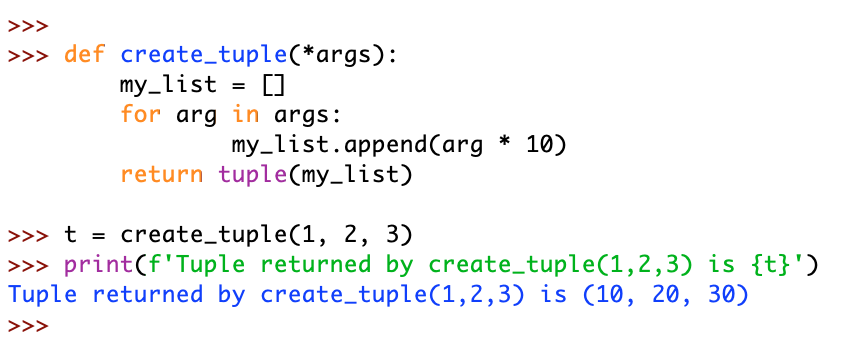
追加の参考文献:Pythonの*argsと**kwargsについて
Pythonの関数が別の関数を返す。
返り値の文から、関数を返すことも可能です。これはカリー化と似ています。カリー化は、複数の引数を受け取る関数の評価を、単一の引数を持つ関数のシーケンスの評価に変換する技法です。
def get_cuboid_volume(h):
def volume(l, b):
return l * b * h
return volume
volume_height_10 = get_cuboid_volume(10)
cuboid_volume = volume_height_10(5, 4)
print(f'Cuboid(5, 4, 10) volume is {cuboid_volume}')
cuboid_volume = volume_height_10(2, 4)
print(f'Cuboid(2, 4, 10) volume is {cuboid_volume}')
出力:
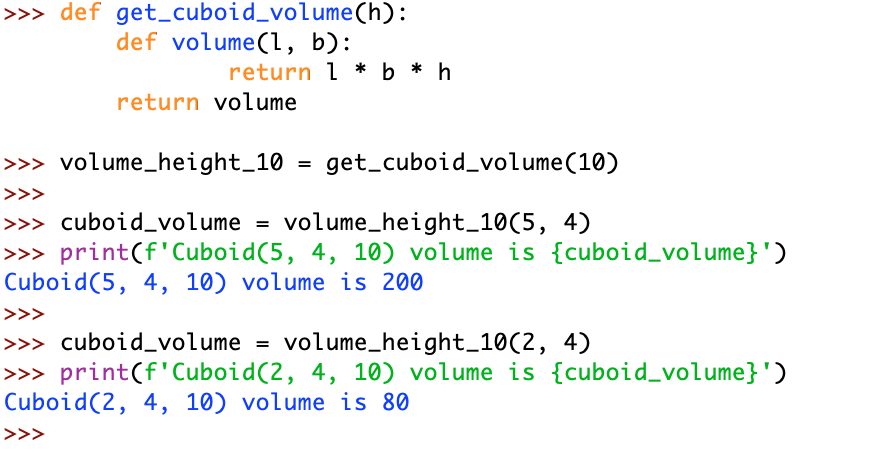
外部関数を返すPythonの関数。
私たちは、関数の外で定義された関数もreturn文で返すことができます。
def outer(x):
return x * 10
def my_func():
return outer
output_function = my_func()
print(type(output_function))
output = output_function(5)
print(f'Output is {output}')
出力する。
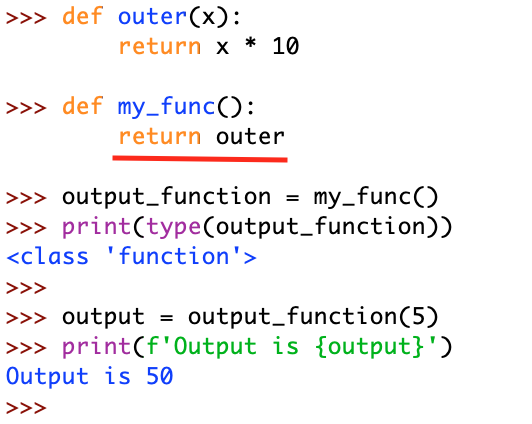
Pythonで複数の値を返す。
関数から複数の値を返したい場合は、要件に応じてタプル、リスト、または辞書オブジェクトを返すことができます。ただし、膨大な数の値を返す必要がある場合は、シーケンスの使用はリソースを多く消費する処理になります。その場合、yieldを使用して一つずつ複数の値を返すことができます。
def multiply_by_five(*args):
for arg in args:
yield arg * 5
a = multiply_by_five(4, 5, 6, 8)
print(a)
# showing the values
for i in a:
print(i)
出力する
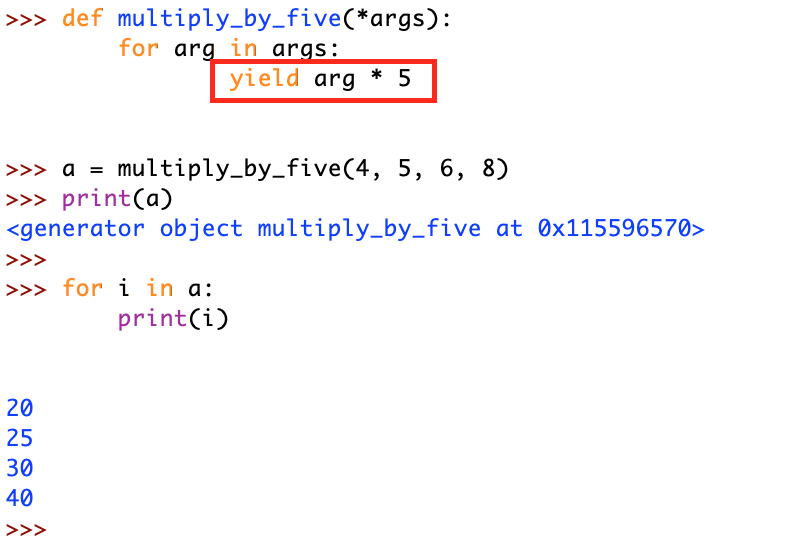
要約
Pythonのreturn文は、関数の出力を返すために使用されます。また、別の関数から関数自体を返すことも学びました。さらに、式は評価され、その結果が関数から返されます。
私たちのGitHubリポジトリからは、完全なPythonスクリプトとさらにPythonの例をチェックアウトできます。