Pythonのsuper() – Python 3のsuper()
Pythonのsuper()関数は、明示的に親クラスを参照することができます。これは、スーパークラスの関数を呼び出したい場合に継承の際に役立ちます。
Pythonスーパー
Pythonのsuper関数について理解するには、Pythonの継承について知っておく必要があります。Pythonの継承では、サブクラスはスーパークラスから継承します。Pythonのsuper()関数を使用すると、スーパークラスを暗黙的に参照することができます。したがって、Pythonのsuperは私たちの課題をより簡単で快適にします。サブクラスからスーパークラスを参照する際に、スーパークラスの名前を明示的に書く必要はありません。次のセクションでは、Pythonのsuper関数について説明します。
Pythonにおけるsuper関数の例
(Python ni okeru super kankei no rei)
最初に、私たちがPythonの継承チュートリアルで使用した次のコードを見てみてください。この例のコードでは、親クラスはPersonであり、子クラスはStudentでした。したがって、コードは以下のように表示されます。
class Person:
# initializing the variables
name = ""
age = 0
# defining constructor
def __init__(self, person_name, person_age):
self.name = person_name
self.age = person_age
# defining class methods
def show_name(self):
print(self.name)
def show_age(self):
print(self.age)
# definition of subclass starts here
class Student(Person):
studentId = ""
def __init__(self, student_name, student_age, student_id):
Person.__init__(self, student_name, student_age)
self.studentId = student_id
def get_id(self):
return self.studentId # returns the value of student id
# end of subclass definition
# Create an object of the superclass
person1 = Person("Richard", 23)
# call member methods of the objects
person1.show_age()
# Create an object of the subclass
student1 = Student("Max", 22, "102")
print(student1.get_id())
student1.show_name()
上記の例では、親クラスの関数を以下のように呼び出しました:
Person.__init__(self, student_name, student_age)
以下のように、この部分はPythonのスーパー関数呼び出しで置き換えることができます。
super().__init__(student_name, student_age)
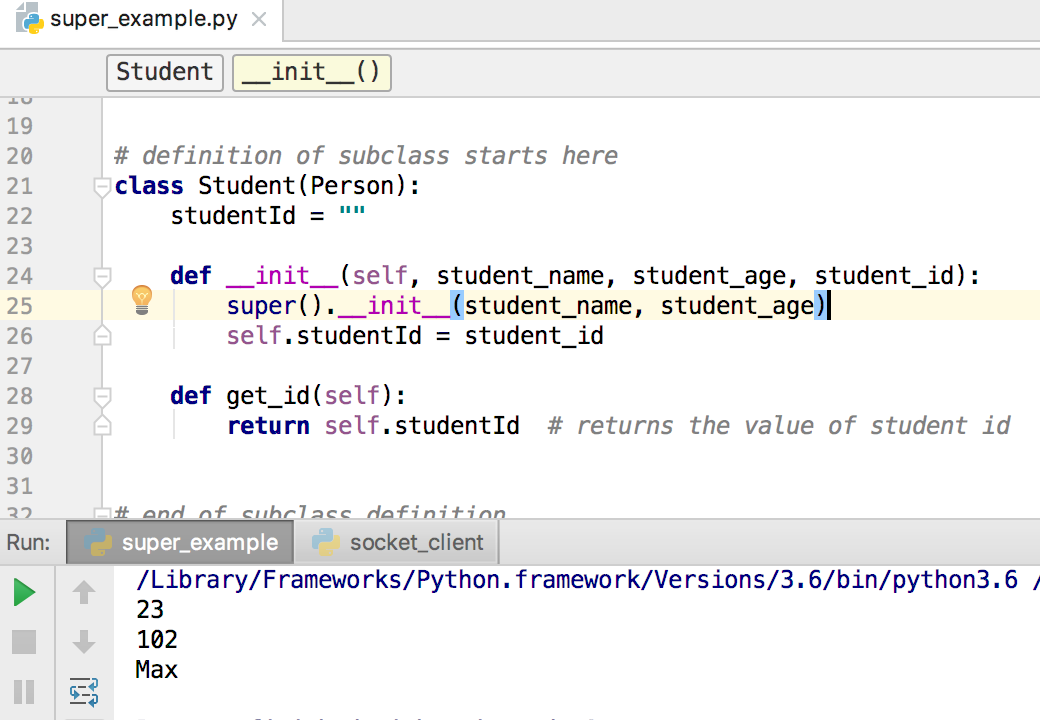
Python 3 のスーパー
上記の構文は、Python 3のスーパー関数用です。もしPython 2.xのバージョンを使用している場合は、若干異なりますので、以下の変更を行う必要があります。
class Person(object):
...
super(Student, self).__init__(student_name, student_age)
最初の変更は、Personのベースクラスとしてobjectを持つことです。これはPython 2.xバージョンではsuper関数を使用する必要があります。さもなければ、以下のエラーが発生します。
Traceback (most recent call last):
File "super_example.py", line 40, in <module>
student1 = Student("Max", 22, "102")
File "super_example.py", line 25, in __init__
super(Student, self).__init__(student_name, student_age)
TypeError: must be type, not classobj
スーパー関数自体の構文の二番目の変更です。Python 3 のスーパー関数は非常に使いやすく、構文も見やすくなっています。
Pythonのマルチレベル継承を持つスーパーファンクション
以前に述べたように、Pythonのsuper()関数は、スーパークラスを暗黙的に参照できるようにします。しかし、多層継承の場合、super()はどのクラスを参照するのでしょうか?実は、Pythonのsuper()は常に直接のスーパークラスを参照します。また、Pythonのsuper()関数は、__init__()関数だけでなく、スーパークラスの他のすべての関数を呼び出すこともできます。それでは、以下の例を見てみましょう。
class A:
def __init__(self):
print('Initializing: class A')
def sub_method(self, b):
print('Printing from class A:', b)
class B(A):
def __init__(self):
print('Initializing: class B')
super().__init__()
def sub_method(self, b):
print('Printing from class B:', b)
super().sub_method(b + 1)
class C(B):
def __init__(self):
print('Initializing: class C')
super().__init__()
def sub_method(self, b):
print('Printing from class C:', b)
super().sub_method(b + 1)
if __name__ == '__main__':
c = C()
c.sub_method(1)
では、上記のPython 3の多段継承を使用したスーパー例の出力を見てみましょう。
Initializing: class C
Initializing: class B
Initializing: class A
Printing from class C: 1
Printing from class B: 2
Printing from class A: 3
ですので、出力から明らかにわかるように、まずクラスCの__init__()関数が呼び出され、その後にクラスB、さらにクラスAが呼び出されました。同様のことがsub_method()関数を呼び出す際にも起こりました。
なぜPythonのスーパー関数が必要なのか。
もしJava言語の以前の経験があるなら、ベースクラスはそこでもスーパーオブジェクトとして呼ばれることを知っているはずです。ですので、この概念は実際にはプログラマにとって便利です。ただし、Pythonでもプログラマはスーパークラスの名前を参照するための機能を保持しています。そして、もしプログラムに多重継承が含まれている場合、このsuper()関数は役に立ちます。以上がPythonのsuper関数についての説明です。このトピックを理解していただければと思います。ご質問はコメントボックスをご利用ください。
私達のGitHubのリポジトリから、完全なPythonのスクリプトや他のPythonの例をチェックアウトすることができます。
参考:公式ドキュメント