在C++中的二维数组
简介
在C++中,二维数组是多维数组的最简单形式。它可以被看作是数组的数组。下面的图像描述了一个二维数组。
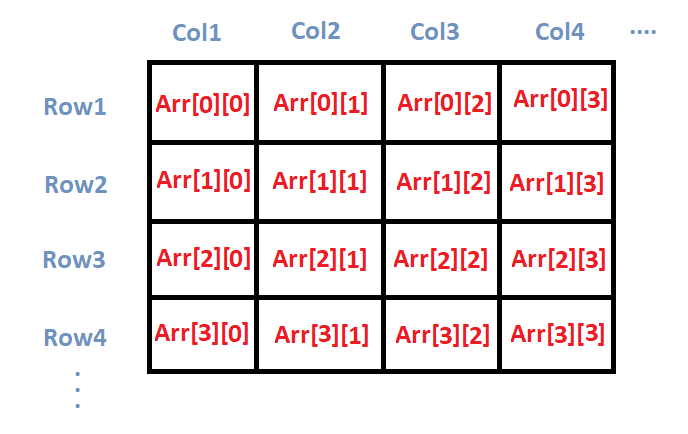
二维数组也被称为矩阵。它可以是任何类型的,如整数、字符、浮点数等,取决于初始化方式。在下一部分中,我们将讨论如何初始化二维数组。
在C++中初始化一个二维数组
那么,在C++中我们如何初始化二维数组呢?就这样简单:
int arr[4][2] = {
{1234, 56},
{1212, 33},
{1434, 80},
{1312, 78}
} ;
所以,如你所见,我们初始化了一个有4行2列的二维数组arr作为一个数组的数组。数组的每个元素又是一个整数数组。
我们也可以通过以下方式初始化一个二维数组。
int arr[4][2] = {1234, 56, 1212, 33, 1434, 80, 1312, 78};
在这种情况下,arr 是一个有4行2列的二维数组。
在C++中打印一个二维数组
我们已经完成了一个二维数组的初始化,现在如果没有实际打印出来,我们无法确认是否初始化正确。
在许多情况下,我们可能需要在进行一些操作后打印结果的二维数组。那么我们该怎么做呢?
以下代码向我们展示了如何实现这一点。
#include<iostream>
using namespace std;
main( )
{
int arr[4][2] = {
{ 10, 11 },
{ 20, 21 },
{ 30, 31 },
{ 40, 41 }
} ;
int i,j;
cout<<"Printing a 2D Array:\n";
for(i=0;i<4;i++)
{
for(j=0;j<2;j++)
{
cout<<"\t"<<arr[i][j];
}
cout<<endl;
}
}
输出:
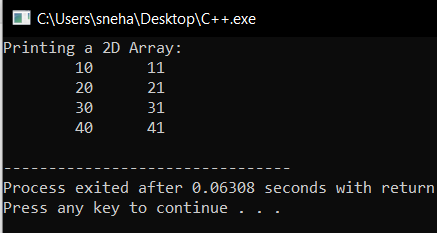
在上面的代码中,
- We firstly initialize a 2D array, arr[4][2] with certain values,
- After that, we try to print the respective array using two for loops,
- the outer for loop iterates over the rows, while the inner one iterates over the columns of the 2D array,
- So, for each iteration of the outer loop, i increases and takes us to the next 1D array. Also, the inner loop traverses over the whole 1D array at a time,
- And accordingly, we print the individual element arr[ i ][ j ].
用户输入2D数组元素
我们之前看到了如何使用预定义的值初始化一个二维数组。但是我们也可以让用户进行输入。让我们看一下如何实现。
#include<iostream>
using namespace std;
main( )
{
int s[2][2];
int i, j;
cout<<"\n2D Array Input:\n";
for(i=0;i<2;i++)
{
for(j=0;j<2;j++)
{
cout<<"\ns["<<i<<"]["<<j<<"]= ";
cin>>s[i][j];
}
}
cout<<"\nThe 2-D Array is:\n";
for(i=0;i<2;i++)
{
for(j=0;j<2;j++)
{
cout<<"\t"<<s[i][j];
}
cout<<endl;
}
}
输出:
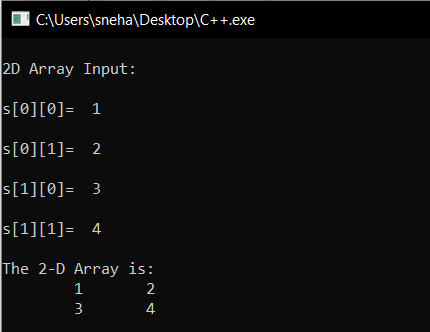
对于以上代码,我们声明一个2X2的二维数组s。使用两个嵌套的for循环,我们遍历数组的每个元素并获取相应的用户输入。通过这种方式,整个数组被填充,并且我们打印出来以查看结果。
在C++中使用二维数组进行矩阵相加。
作为一个例子,让我们看看如何使用二维数组进行矩阵相加并打印结果。
#include<iostream>
using namespace std;
main()
{
int m1[5][5], m2[5][5], m3[5][5];
int i, j, r, c;
cout<<"Enter the no.of rows of the matrices to be added(max 5):";
cin>>r;
cout<<"Enter the no.of columns of the matrices to be added(max 5):";
cin>>c;
cout<<"\n1st Matrix Input:\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
cout<<"\nmatrix1["<<i<<"]["<<j<<"]= ";
cin>>m1[i][j];
}
}
cout<<"\n2nd Matrix Input:\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
cout<<"\nmatrix2["<<i<<"]["<<j<<"]= ";
cin>>m2[i][j];
}
}
cout<<"\nAdding Matrices...\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
m3[i][j]=m1[i][j]+m2[i][j];
}
}
cout<<"\nThe resultant Matrix is:\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
cout<<"\t"<<m3[i][j];
}
cout<<endl;
}
}
输出:
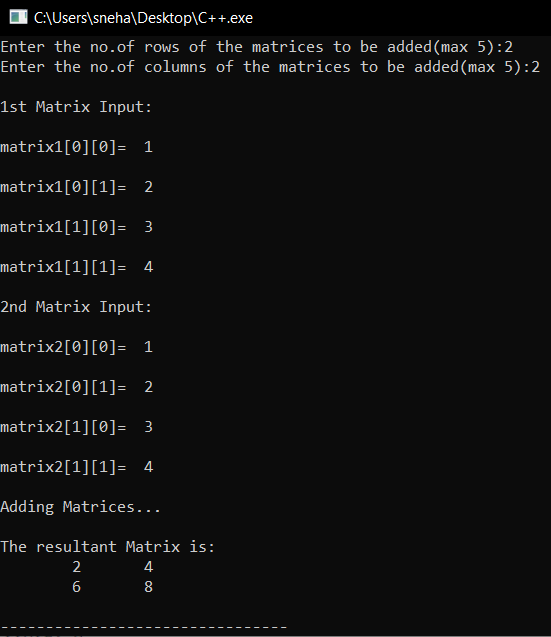
这里
- We take two matrices m1 and m2 with a maximum of 5 rows and 5 columns. And another matrix m3 in which we are going to store the result,
- As user inputs, we took the number of rows and columns for both the matrices. Since we are performing matrix addition, the number of rows and columns should be the same for both the matrices,
- After that, we take both the matrices as user inputs, again using nested for loops,
- At this point, we have both the matrices m1 and m2,
- then we traverse through the m3 matrix, using two for loops and update the respective elements m3[ i ][ j ] by the value of m1[i][j]+m2[i][j]. In this way, by the end of the outer for loop, we get our desired matrix,
- At last, we print out the resultant matrix m3.
指向C++中的2D数组的指针
如果我们可以有一个指向整数的指针,一个指向浮点数的指针,一个指向字符的指针,那么难道我们不能有一个指向数组的指针吗?当然可以。下面的程序展示了如何构建和使用它。
#include<iostream>
using namespace std;
/* Usage of pointer to an array */
main( )
{
int s[5][2] = {
{1, 2},
{1, 2},
{1, 2},
{1, 2}
} ;
int (*p)[2] ;
int i, j;
for (i = 0 ; i <= 3 ; i++)
{
p=&s[i];
cout<<"Row"<<i<<":";
for (j = 0; j <= 1; j++)
cout<<"\t"<<*(*p+j);
cout<<endl;
}
}
结果:
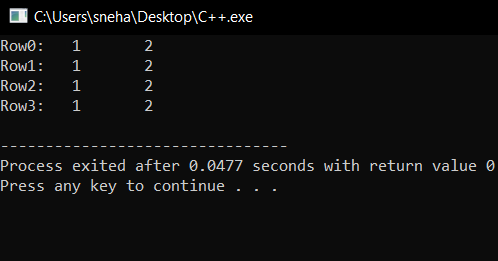
这里,
- In the above code, we try to print a 2D array using pointers,
- As we earlier did, at first we initialize the 2D array, s[5][2]. And also a pointer (*p)[2], where p is a pointer which stores the address of an array with 2 elements,
- As we already said, we can break down a 2D array as an array of arrays. So in this case, s is actually an array with 5 elements, which further are actually arrays with 2 elements for each row.
- We use a for loop to traverse over these 5 elements of the array, s. For each iteration, we assign p with the address of s[i],
- Further, the inner for loop prints out the individual elements of the array s[i] using the pointer p. Here, (*p + j) gives us the address of the individual element s[i][j], so using *(*p+j) we can access the corresponding value.
将一个二维数组传递给函数
在这一节中,我们将学习如何将一个二维数组传递给任何函数,并访问相应的元素。在下面的代码中,我们将数组a传递给show()和print()两个函数,这两个函数将打印出传递的二维数组。
#include<iostream>
using namespace std;
void show(int (*q)[4], int row, int col)
{
int i, j ;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
cout<<"\t"<<*(*(q + i)+j);
cout<<"\n";
}
cout<<"\n";
}
void print(int q[][4], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
cout<<"\t"<<q[i][j];
cout<<"\n";
}
cout<<"\n";
}
int main()
{
int a[3][4] = { 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21} ;
show (a, 3, 4);
print (a, 3, 4);
return 0;
}
输出:需要用中文原生地进行改述,只需要一种选项:
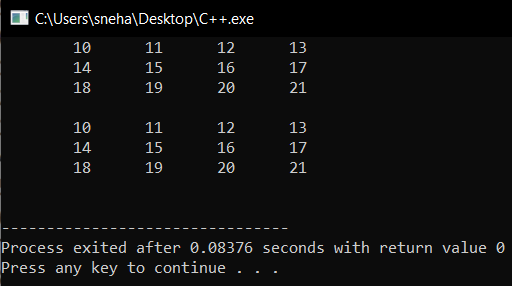
这里
- In the show( ) function we have defined q to be a pointer to an array of 4 integers through the declaration int (*q)[4],
- q holds the base address of the zeroth 1-D array
- This address is then assigned to q, an int pointer, and then using this pointer all elements of the zeroth 1D array are accessed.
- Next time through the loop when i takes a value 1, the expression q+i fetches the address of the first 1-D array. This is because q is a pointer to the zeroth 1-D array and adding 1 to it would give us the address of the next 1-D array. This address is once again assigned to q and using it all elements of the next 1-D array are accessed
- In the second function print(), the declaration of q looks like this: int q[][4] ,
- This is same as int (*q )[4], where q is a pointer to an array of 4 integers. The only advantage is that we can now use the more familiar expression q[i][j] to access array elements. We could have used the same expression in show() as well but for better understanding of the use of pointers, we use pointers to access each element.
结论
因此,在本文中,我们讨论了C++中的二维数组,我们如何执行各种操作以及其在矩阵加法中的应用。如有任何进一步的问题,请随时在评论中提问。
参考资料
- https://en.wikipedia.org/wiki/Array_data_structure
- /community/tutorials/arrays-in-c