如何使用Python读取大型文本文件
Python的文件对象提供了多种读取文本文件的方法。其中一种常用的方式是使用readlines()方法,它会返回文件中所有行的列表。然而,这种方法不适用于读取大型文本文件,因为整个文件内容会被加载到内存中。
在Python中读取大型文本文件
我们可以使用文件对象作为迭代器。迭代器将逐行逐个返回,可以进行处理。这样不会将整个文件读入内存,适合在Python中读取大文件。以下是将大文件视为迭代器来读取的代码片段。
import resource
import os
file_name = "/Users/scdev/abcdef.txt"
print(f'File Size is {os.stat(file_name).st_size / (1024 * 1024)} MB')
txt_file = open(file_name)
count = 0
for line in txt_file:
# we can process file line by line here, for simplicity I am taking count of lines
count += 1
txt_file.close()
print(f'Number of Lines in the file is {count}')
print('Peak Memory Usage =', resource.getrusage(resource.RUSAGE_SELF).ru_maxrss)
print('User Mode Time =', resource.getrusage(resource.RUSAGE_SELF).ru_utime)
print('System Mode Time =', resource.getrusage(resource.RUSAGE_SELF).ru_stime)
当我们运行这个程序时,所产生的输出是:
File Size is 257.4920654296875 MB
Number of Lines in the file is 60000000
Peak Memory Usage = 5840896
User Mode Time = 11.46692
System Mode Time = 0.09655899999999999
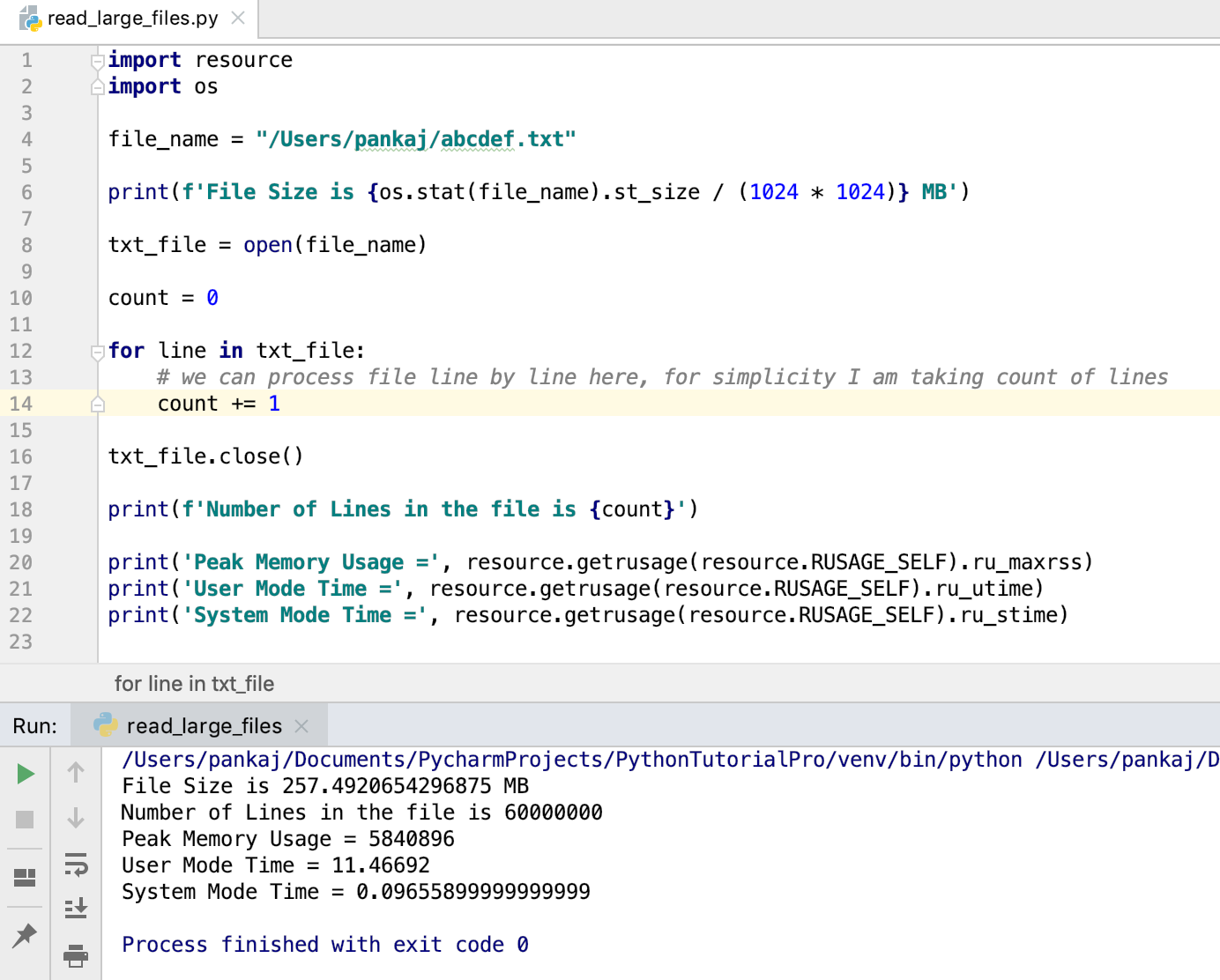
- I am using os module to print the size of the file.
- The resource module is used to check the memory and CPU time usage of the program.
我们还可以使用with语句打开文件。在这种情况下,我们不需要显式关闭文件对象。
with open(file_name) as txt_file:
for line in txt_file:
# process the line
pass
如果这个大文件没有行数,怎么办?
当大文件内容被分割为多行时,上述代码将运行得很好。但是,如果单行中有大量数据,它将使用大量内存。在这种情况下,我们可以将文件内容读入缓冲区并进行处理。
with open(file_name) as f:
while True:
data = f.read(1024)
if not data:
break
print(data)
上述代码将文件数据读入一个大小为1024字节的缓冲区。然后我们将其打印到控制台。当整个文件被读取完毕时,数据将变为空,并且break语句将终止while循环。该方法还可用于读取二进制文件,如图片、PDF、Word文档等。以下是一个简单的代码片段,用于复制文件。
with open(destination_file_name, 'w') as out_file:
with open(source_file_name) as in_file:
for line in in_file:
out_file.write(line)
参考:StackOverflow问题