在Selenium Chrome Driver上执行测试
Chrome浏览器通过使用一个称为ChromeDriver.exe的可执行文件来实现WebDriver协议。该可执行文件在您的系统上启动一个服务器,所有的测试将通过该服务器与其通信以运行测试。在本文中,我们将学习如何下载最新版本的Selenium ChromeDriver。
- How to setup Selenium ChromeDriver in multiple ways
下载Selenium ChromeDriver
首先,我们需要下载最新版本的ChromeDriver,主要是因为它支持最新版本的Chrome,并且包含了所有的错误修复。以下是下载ChromeDriver的步骤:
第一步:前往Chromium官方网站,根据你的操作系统下载最新版本的ChromeDriver。
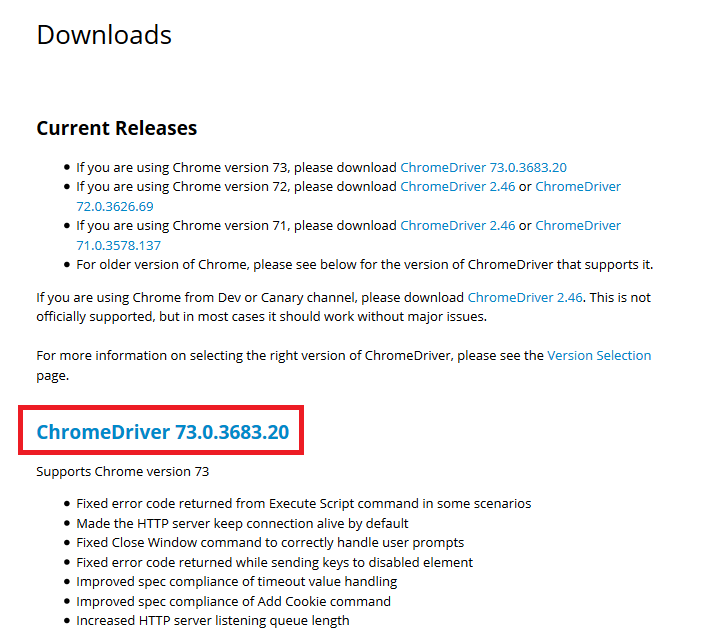
- Step 2: Click on ChromeDriver 73.0.3683.20 link. You will be navigated to ChromeDriver download page which contains ChromeDriver for Linux, Mac and Windows operating systems.
Note: Here we are working on Windows Operating system, we need to download the corresponding Chrome driver of Windows version. If your Operating System is Linux or Mac then you need to download the corresponding Chrome driver.
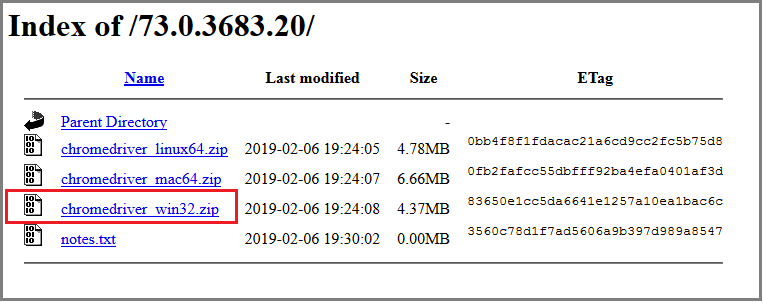
- Step 3: Click on chromedriver_win32.zip to download ChromeDriver for Windows.
- Step 4: Once the zip file is downloaded, you can unzip it to retrieve chromedriver.exe. Note the location where you extracted the ChromeDriver. Location will be later used to instantiate the driver.
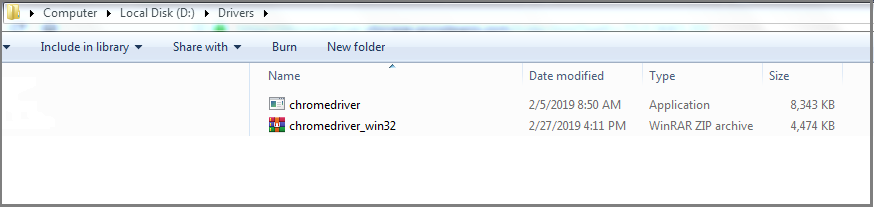
使用Selenium WebDriver启动Chrome浏览器
启动Chrome浏览器驱动与启动其他驱动一样容易。WebDriver = new ChromeDriver();
package com.Olivia.selenium.Chrome;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ChromeDriver {
public static void main(String[] args) {
WebDriver driver= new ChromeDriver();
driver.get("https://journaldev.com");
}
}
当您运行上述程序时,我们会得到一个叫做java.lang.IllegalStateException的异常,表示必须通过webdriver.chrome.driver设置驱动程序可执行文件的路径。为了解决上述问题,我们需要下载ChromeDriver以便使用我们在Chrome上编写的Selenium命令。每个浏览器都有相应的驱动程序,Chrome的驱动程序是ChromeDriver。Selenium命令将由ChromeDriver解释并在Chrome上执行。
初始化ChromeDriver的不同方法
初始化ChromeDriver有两种方法- 使用Webdriver.Chrome.Driver
- Use Environment Variables
方法一:使用Webdriver.chrome.driver系统属性
设置系统属性的代码
System.setProperty(“webdriver.chrome.driver”,“Path to chromedriver.exe”);
启动ChromeDriver的完整程序将如下所示:
package com.Olivia.selenium.Chrome;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ChromeDriver {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver","D:\\Drivers\\chromedriver.exe");
WebDriver driver= new ChromeDriver();
driver.get("https://journaldev.com");
String PageTitle = driver.getTitle();
System.out.println("Page Title is:" + PageTitle);
driver.close();
}
}
当你运行以上的程序时,你会注意到Journaldev.com在新的Chrome窗口中打开,而且它会在控制台中打印出网站的标题。
方法2:在Windows环境变量中设置ChromeDriver路径。
- Step 1: Go to My Computer and Right click to get the context menu.
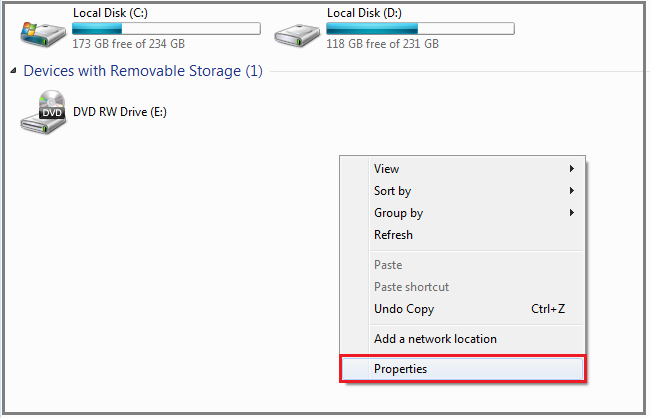
- Step 2: Click on the Change Setting on the opened window.
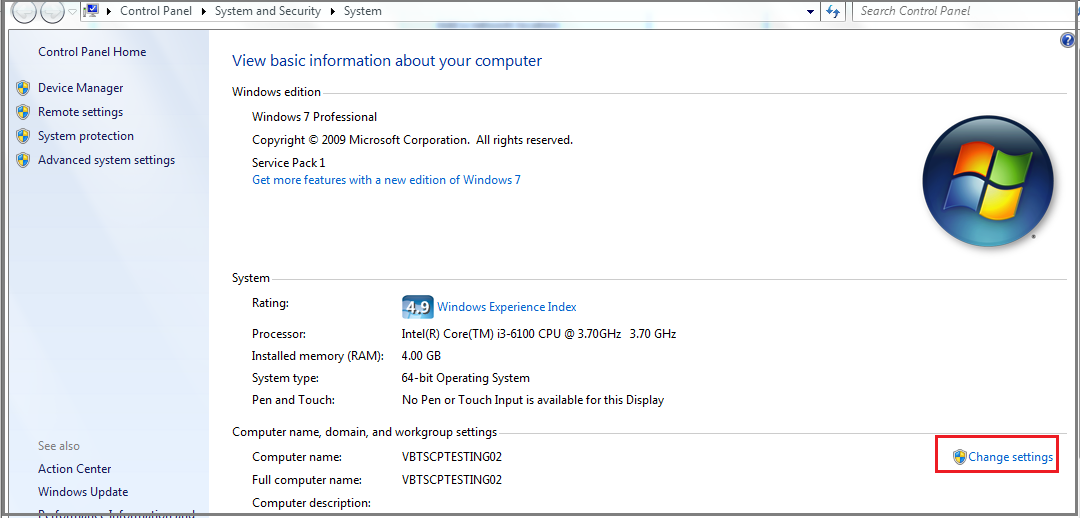
- Step 3: Click on Advance tab and click on Environment Variables.
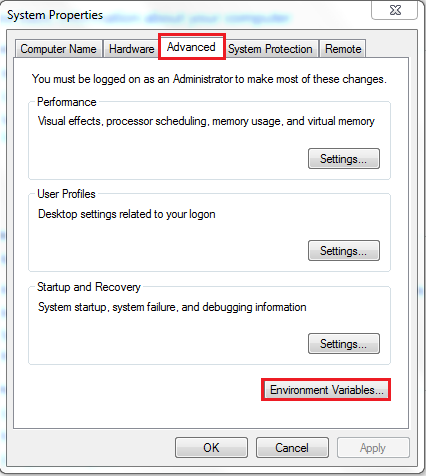
- Step 4: Select Path under System Variables and click on Edit.
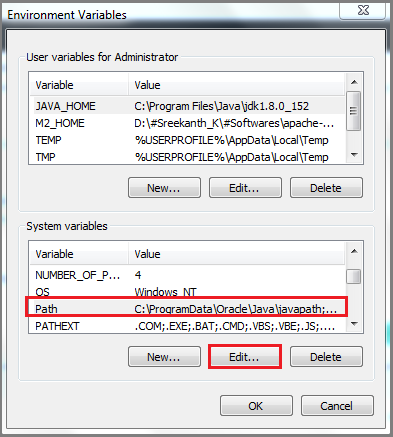
- Step 5: At the end of the string use semicolon and paste the path of the ChromeDriver. On my machine my ChromeDriver exe resides in D:\Drivers\
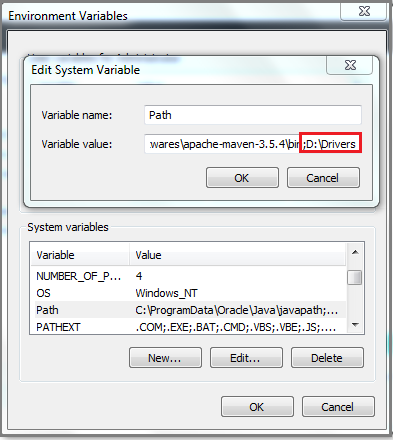
注意:一旦路径设置好了,你就不需要每次在脚本中设置系统属性。你的脚本可以在没有系统属性代码的情况下正常工作。启动ChromeDriver的完整程序如下:
package com.Olivia.selenium.Chrome;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromefoxDriver;
public class ChromeDriver {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://journaldev.com");
String PageTitle = driver.getTitle();
System.out.println("Page Title is:" + PageTitle);
driver.close();
}
}
当你运行上述程序时,你的脚本将在没有系统属性代码的情况下正常工作,你会注意到Journaldev.com在新的Chrome窗口中被打开,并且它会在控制台中打印出网站标题。