S型激活函数 – Python 实现
在本教程中,我们将学习Sigmoid激活函数。Sigmoid函数始终返回介于0和1之间的输出。
在完成本教程后,你将会了解到:
- What is an activation function?
- How to implement the sigmoid function in python?
- How to plot the sigmoid function in python?
- Where do we use the sigmoid function?
- What are the problems caused by the sigmoid activation function?
- Better alternatives to the sigmoid activation.
什么是激活函数?
激活函数是控制神经网络输出的数学函数。激活函数有助于确定神经元是否应该被激活。
一些常见的激活函数包括:
- Binary Step
- Linear
- Sigmoid
- Tanh
- ReLU
- Leaky ReLU
- Softmax
激活函数负责为神经网络模型的输出引入非线性。如果没有激活函数,神经网络只是一个线性回归模型。
神经网络输出的数学公式为:

在这个教程中,我们将专注于sigmoid激活函数。这个函数源自数学中的sigmoid函数。
让我们从讨论函数的公式开始。 de .)
Sigmoid激活函数的公式
数学上可以把Sigmoid激活函数表示为:
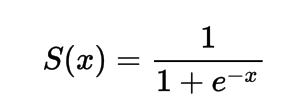
你可以看到分母总是大于1,因此输出将始终在0和1之间。
在Python中实现Sigmoid激活函数。
在这一部分中,我们将学习如何在Python中实现Sigmoid激活函数。
我们可以在Python中定义函数为:
import numpy as np
def sig(x):
return 1/(1 + np.exp(-x))
让我们尝试在一些输入上运行这个函数。
import numpy as np
def sig(x):
return 1/(1 + np.exp(-x))
x = 1.0
print('Applying Sigmoid Activation on (%.1f) gives %.1f' % (x, sig(x)))
x = -10.0
print('Applying Sigmoid Activation on (%.1f) gives %.1f' % (x, sig(x)))
x = 0.0
print('Applying Sigmoid Activation on (%.1f) gives %.1f' % (x, sig(x)))
x = 15.0
print('Applying Sigmoid Activation on (%.1f) gives %.1f' % (x, sig(x)))
x = -2.0
print('Applying Sigmoid Activation on (%.1f) gives %.1f' % (x, sig(x)))
输出:结果
Applying Sigmoid Activation on (1.0) gives 0.7
Applying Sigmoid Activation on (-10.0) gives 0.0
Applying Sigmoid Activation on (0.0) gives 0.5
Applying Sigmoid Activation on (15.0) gives 1.0
Applying Sigmoid Activation on (-2.0) gives 0.1
使用Python绘制Sigmoid激活函数的图表。
为了绘制S型激活函数,我们将使用Numpy库。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-10, 10, 50)
p = sig(x)
plt.xlabel("x")
plt.ylabel("Sigmoid(x)")
plt.plot(x, p)
plt.show()
产出/输出
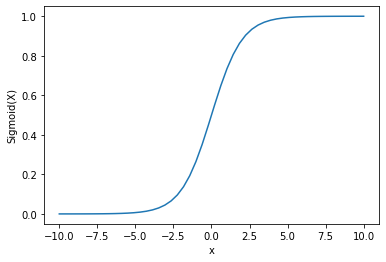
我们可以看到输出在0和1之间。
由于概率始终在0和1之间,因此S型函数通常用于预测概率。
在Sigmoid函数中的一个缺点是在末端区域,Y值对X值的变化反应非常微弱。
这导致了一个被称为梯度消失问题的难题。
梯度消失会降低学习过程的速度,因此是不可取的。
让我们讨论一些能克服这个问题的替代方案。
ReLu 激活函数。
解决梯度消失问题的更好选择是使用ReLu激活函数。
如果输入为负数,则ReLu激活函数返回0;否则返回输入本身。
数学上它的表示方法是:

你可以用Python来实现,步骤如下:
def relu(x):
return max(0.0, x)
让我们看看它在一些输入上的运行方式。
def relu(x):
return max(0.0, x)
x = 1.0
print('Applying Relu on (%.1f) gives %.1f' % (x, relu(x)))
x = -10.0
print('Applying Relu on (%.1f) gives %.1f' % (x, relu(x)))
x = 0.0
print('Applying Relu on (%.1f) gives %.1f' % (x, relu(x)))
x = 15.0
print('Applying Relu on (%.1f) gives %.1f' % (x, relu(x)))
x = -20.0
print('Applying Relu on (%.1f) gives %.1f' % (x, relu(x)))
输出:
Applying Relu on (1.0) gives 1.0
Applying Relu on (-10.0) gives 0.0
Applying Relu on (0.0) gives 0.0
Applying Relu on (15.0) gives 15.0
Applying Relu on (-20.0) gives 0.0
ReLu的问题在于负输入的梯度为零。
这再次引出了负输入的梯度消失(零梯度)的问题。
为了解决这个问题,我们还有另一种选择,被称为泄露线性整流激活函数(Leaky ReLu)。
泄漏线性整流激活函数
通过给负数输入一个极小的线性成分,带有泄漏的ReLU解决了负数梯度为零的问题。
数学上我们可以将其定义为: (Shù xué qí
f(x)= 0.01x, x<0
= x, x>=0
你可以用Python实现它。
def leaky_relu(x):
if x>0 :
return x
else :
return 0.01*x
x = 1.0
print('Applying Leaky Relu on (%.1f) gives %.1f' % (x, leaky_relu(x)))
x = -10.0
print('Applying Leaky Relu on (%.1f) gives %.1f' % (x, leaky_relu(x)))
x = 0.0
print('Applying Leaky Relu on (%.1f) gives %.1f' % (x, leaky_relu(x)))
x = 15.0
print('Applying Leaky Relu on (%.1f) gives %.1f' % (x, leaky_relu(x)))
x = -20.0
print('Applying Leaky Relu on (%.1f) gives %.1f' % (x, leaky_relu(x)))
产出:
Applying Leaky Relu on (1.0) gives 1.0
Applying Leaky Relu on (-10.0) gives -0.1
Applying Leaky Relu on (0.0) gives 0.0
Applying Leaky Relu on (15.0) gives 15.0
Applying Leaky Relu on (-20.0) gives -0.2
结论 (jié
这个教程是关于Sigmoid激活函数的。我们学习了如何在Python中实现和绘制该函数。