Python 中的 super() – Python 3 中的 super()
Python的super()函数允许我们明确地引用父类。在继承的情况下,当我们想要调用父类的函数时,它非常有用。
Python的超级
要了解 Python 的 super() 函数,你必须了解 Python 的继承。在 Python 的继承中,子类会继承自父类。Python 的 super() 函数可以隐式地引用父类。因此,Python 的 super() 函数使得我们的任务更加简单和舒适。当从子类引用父类时,我们不需要显式地写出父类的名称。下面我们将讨论 Python 的 super() 函数。
Python super 函数示例
Python super 函数示例
首先,只需看一下我们在Python继承教程中使用的以下代码。在那个示例代码中,超类是Person,子类是Student。所以代码如下所示。
class Person:
# initializing the variables
name = ""
age = 0
# defining constructor
def __init__(self, person_name, person_age):
self.name = person_name
self.age = person_age
# defining class methods
def show_name(self):
print(self.name)
def show_age(self):
print(self.age)
# definition of subclass starts here
class Student(Person):
studentId = ""
def __init__(self, student_name, student_age, student_id):
Person.__init__(self, student_name, student_age)
self.studentId = student_id
def get_id(self):
return self.studentId # returns the value of student id
# end of subclass definition
# Create an object of the superclass
person1 = Person("Richard", 23)
# call member methods of the objects
person1.show_age()
# Create an object of the subclass
student1 = Student("Max", 22, "102")
print(student1.get_id())
student1.show_name()
在上面的例子中,我们将父类函数称为:
Person.__init__(self, student_name, student_age)
我们可以用Python的超级函数调用来替换这个。
super().__init__(student_name, student_age)
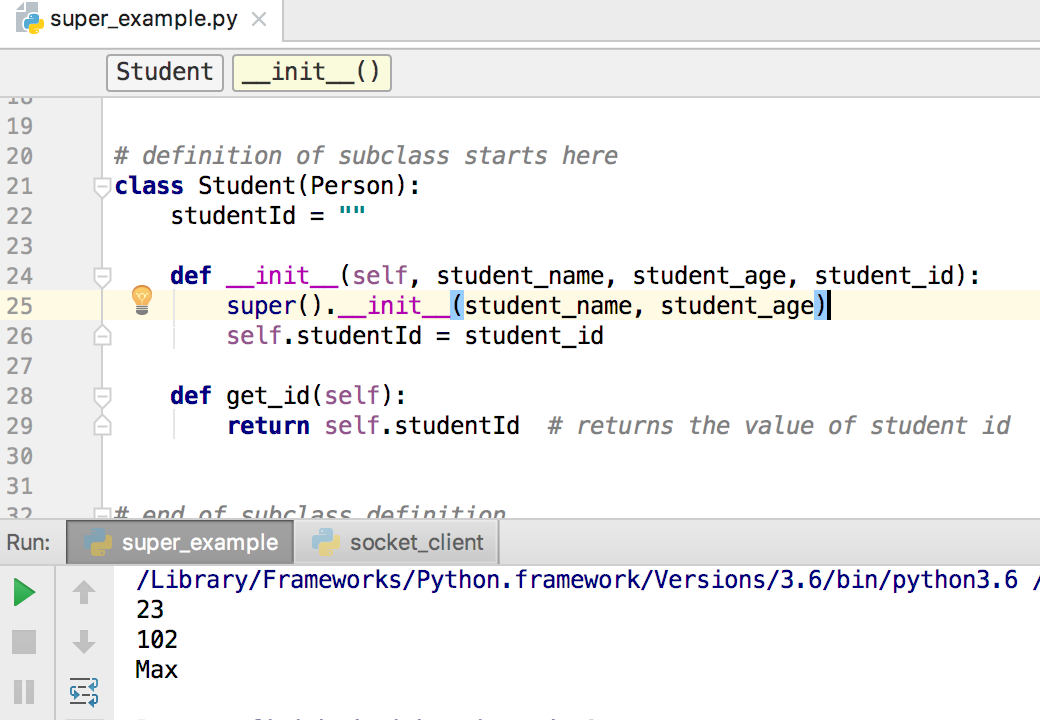
Python 3 的超级
请注意,上述语法适用于Python 3中的super函数。如果您使用的是Python 2.x版本,则会有些许差异,您需要进行以下更改:
class Person(object):
...
super(Student, self).__init__(student_name, student_age)
首先的变化是将对象作为Person的基类。在Python 2.x版本中,使用super函数是必需的。否则,您将会得到以下错误。
Traceback (most recent call last):
File "super_example.py", line 40, in <module>
student1 = Student("Max", 22, "102")
File "super_example.py", line 25, in __init__
super(Student, self).__init__(student_name, student_age)
TypeError: must be type, not classobj
超函数本身的语法的第二个变化。如你所见,Python 3 的超函数使用起来更加简便,语法也更加清晰。
Python超级函数与多级继承
正如我们先前所述,Python的super()函数允许我们隐式地引用超类。但在多级继承的情况下,它会引用哪个类?嗯,Python的super()函数将始终引用最近的超类。此外,Python的super()函数不仅可以引用__init__()函数,还可以调用超类的所有其他函数。因此,在以下示例中,我们将看到这一点。
class A:
def __init__(self):
print('Initializing: class A')
def sub_method(self, b):
print('Printing from class A:', b)
class B(A):
def __init__(self):
print('Initializing: class B')
super().__init__()
def sub_method(self, b):
print('Printing from class B:', b)
super().sub_method(b + 1)
class C(B):
def __init__(self):
print('Initializing: class C')
super().__init__()
def sub_method(self, b):
print('Printing from class C:', b)
super().sub_method(b + 1)
if __name__ == '__main__':
c = C()
c.sub_method(1)
我们来看看上述Python 3超级例子在多级继承中的输出。
Initializing: class C
Initializing: class B
Initializing: class A
Printing from class C: 1
Printing from class B: 2
Printing from class A: 3
所以,从输出中我们可以清晰地看到,首先调用了类C的__init__()方法,然后调用了类B,然后再调用了类A。调用sub_method()方法时也发生了类似的情况。
为什么我们需要Python的超级函数?
如果你在Java编程语言方面有过经验,那么你应该知道在那里基类也被称为super对象。所以对于程序员来说,这个概念实际上是有用的。然而,Python也保留了给程序员使用超类名称来引用它们的功能。而且,如果你的程序包含多层继承,那么super()函数对你来说是有帮助的。这就是关于Python super函数的全部内容了。希望你理解了这个主题。请在评论框中提问。
您可以从我们的GitHub存储库中查看完整的Python脚本和更多Python示例。
参考文献:官方文档