Python 关键字和标识符(更新版)
让我们来谈谈Python的关键字和标识符。最近在这个Python教程中,我们还介绍了适用于初学者的Python安装和设置的完整教程。
Python 关键字
简单来说,Python关键字是被保留的词语。这意味着你不能将它们作为变量、类和函数等实体的名称。
那么你可能会想,这些关键词是用来定义Python语言的语法和结构的。
你应该知道,截至编写本教程时,Python编程语言中有33个关键字。虽然这个数量可能随着时间的推移而有所变化。此外,Python中的关键字是区分大小写的,因此必须按照原样书写。以下是Python编程中所有关键字的列表。
如果你一次性查看所有关键词并尝试弄清楚,你会感到不知所措。 所以现在只需要知道这些是关键词即可。我们将分别学习它们的用途。你可以通过 Python Shell 获取Python关键词列表。
所有Python关键字列表
and | Logical operator |
as | Alias |
assert | For debugging |
break | Break out of Python loops |
class | Used for defining Classes in Python |
continue | Keyword used to continue with the Python loop by skipping the existing |
def | Keyword used for defining a function |
del | Used for deleting objects in Python |
elif | Part of the if-elif-else conditional statement in Python |
else | Same as above |
except | A Python keyword used to catch exceptions |
FALSE | Boolean value |
finally | This keyword is used to run a code snippet when no exceptions occur |
for | Define a Python for loop |
from | Used when you need to import only a specific section of a module |
global | Specify a variable scope as global |
if | Used for defining an “if” condition |
import | Python keyword used to import modules |
in | Checks if specified values are present in an iterable object |
is | This keyword is used to test for equality. |
lambda | Create anonymous functions |
None | The None keyword represents a Null value in PYthon |
nonlocal | Declare a variable with non-local scope |
not | Logical operator to negate a condition |
or | A logical operator used when either one of the conditions needs to be true |
pass | This Python keyword passes and lets the function continue further |
raise | Raises an exception when called with the specified value |
return | Exits a running function and returns the value specified |
TRUE | Boolean value |
try | Part of the try…except statement |
while | Used for defining a Python while loop |
with | Creates a block to make exception handling and file operations easy |
yield | Ends a function and returns a generator object |
以下是一个简单的示例,展示了在Python程序中使用if-else的用法。
var = 1;
if(var==1):
print("odd")
else:
print("even")
当我们运行上述程序时,Python通过固定的关键字和语法,理解了if-else代码块,并进行进一步的处理。
Python标识符是什么?
Python中的标识符是我们给变量、函数、类、模块或其他对象赋予的名称。这意味着每当我们想要给实体起一个名字时,就称之为标识符。
有时候,变量和标识符经常被误解为一样,但实际上它们是不同的。为了明确起见,让我们看看什么是变量。
在Python中,什么是变量?
变量,顾名思义,是指其值随时间可变的东西。实际上,变量就是一个可以存储值的内存位置。稍后我们可以检索这个值并使用它。但是为了实现这一点,我们需要给这个内存位置取一个昵称,以便我们能够引用它。这就是标识符,也就是昵称。
写标识符的规则
在写标识符时有一些规则。但首先你必须知道Python是区分大小写的。这意味着在Python中,Name和name是两个不同的标识符。以下是编写Python标识符的一些规则。
-
- 标识符可以由大写字母、小写字母、数字或下划线(_)的组合构成。因此,myVariable、variable_1和variable_for_print都是有效的Python标识符。
-
- 标识符不能以数字开头。因此,variable1是有效的,而1variable是无效的。
-
- 我们不能在标识符中使用特殊符号如!、#、@、%、$等。
- 标识符的长度可以是任意的。
尽管这些规则对于编写标识符来说是固定的,但还有一些命名约定,并非强制要求,而是遵循的好方法。
-
- 类名的开头是大写字母。所有其他标识符都以小写字母开头。
-
- 以单个下划线作为标识符的开头表示该标识符是私有的。
-
- 如果标识符以两个下划线开头和结尾,则表示该标识符是语言定义的特殊名称。
-
- 虽然 c = 10 是有效的,但写成 count = 10 更有意义,而且即使在很长时间后查看代码时,也更容易理解它的功能。
- 多个单词可以用下划线分隔,例如 this_is_a_variable。
这是一个Python变量的示例程序。
myVariable="hello world"
print(myVariable)
var1=1
print(var1)
var2=2
print(var2)
如果你运行这个程序,输出的结果将会是下面的图片。
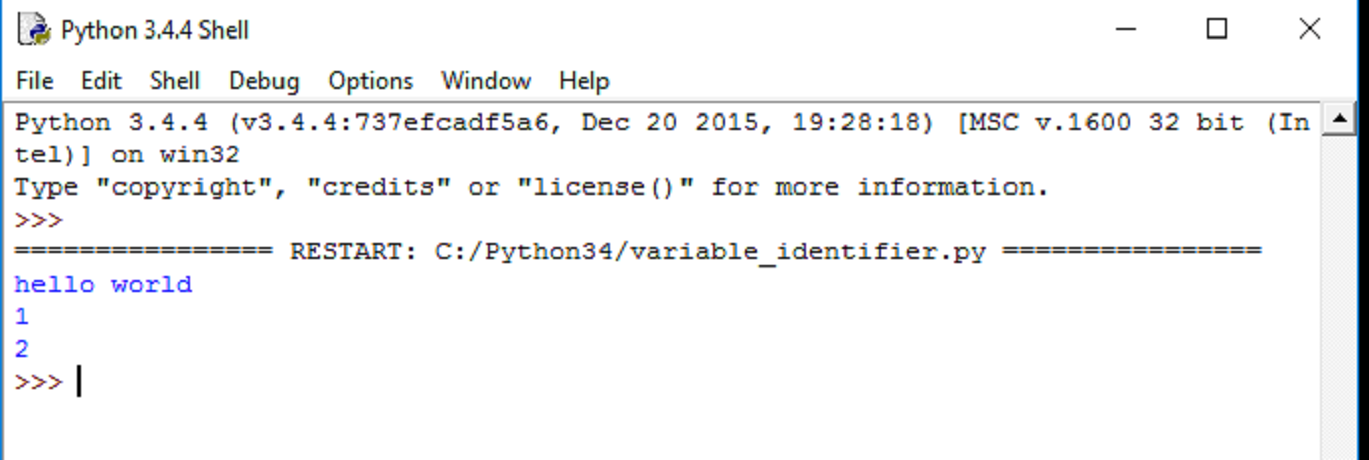
結論
所以,今天就讲到这里。下一节教程中,我们将学习关于Python语句和注释的内容。在那之前,#愉快编程 🙂