Selenium WebDriver = Selenium驱动程序
我们需要浏览器来测试Web应用程序。Selenium自动化浏览器并可以帮助我们自动化不同浏览器上的Web应用程序测试。Selenium API提供了许多类和接口,用于处理不同类型的浏览器和HTML元素。
什么是Selenium WebDriver接口?
Selenium WebDriver是一个定义了一组方法的接口。然而,具体的实现由特定浏览器的类提供。其中一些实现类包括AndroidDriver,ChromeDriver,FirefoxDriver,InternetExplorerDriver,IPhoneDriver,SafariDriver等。WebDriver的主要功能是控制浏览器。它甚至帮助我们选择HTML页面元素并对其执行操作,例如点击,填写表单字段等。
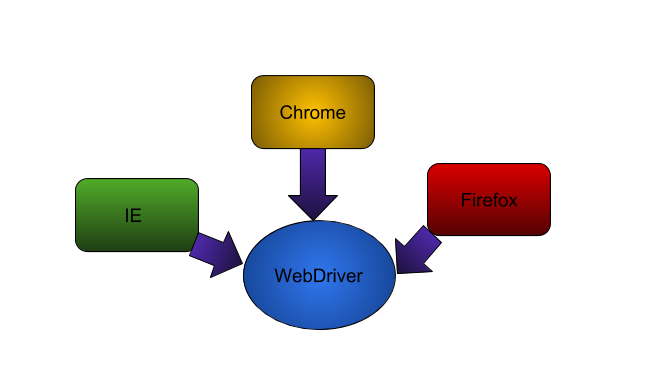
如果我们想在Firefox浏览器中执行您的测试用例,我们必须使用FirefoxDriver类。同样地,如果我们想在Chrome浏览器中执行测试用例,我们必须使用ChromeDriver类。
Selenium WebDriver方法的释义
SearchContext是Selenium API中最高级的接口,它有两个方法 – findElement()和findElements()。Selenium WebDriver接口有许多抽象方法,如get(String url),quit(),close(),getWindowHandle(),getWindowHandles(),getTitle()等。WebDriver还有嵌套接口,如Window、Navigation、Timeouts等。这些嵌套接口用于执行back(),forward()等操作。
Method | Description |
---|---|
get(String url) | This method will launch a new browser and opens the given URL in the browser instance. |
getWindowHandle() | It is used to handle single window i.e. main window. It return type is string. It will returns browser windlw handle from focused browser. |
getWindowHandles() | It is used to handle multiple windows. It return type is Set. It will returns all handles from all opened browsers by Selenium WebDriver. |
close() | This command is used to close the current browser window which is currently in focus. |
quit() | This method will closes all the browsers windows which are currently opened and terminates the WebDriver session. |
getTitle() | This method is used to retrieve the title of the webpage the user currently working on. |
实现WebDriver接口的类列表
WebDriver接口的主要实现类包括ChromeDriver、EdgeDriver、FirefoxDriver、InternetExplorerDriver等。每个驱动器类对应一个浏览器。我们只需创建驱动器类的对象并与其一起使用即可。
Class | Description |
---|---|
ChromeDriver | It helps you to execute Selenium Scripts on Chrome browser. |
FirefoxDriver | It helps you to execute Selenium Scripts on Firefox browser. |
InternetExplorerDriver | It helps you to execute Selenium Scripts on InternetExplorer browser. |
WebElement命令列表
Selenium的WebElement代表一个HTML元素。我们可以使用findElement()方法获得WebElement的实例,然后执行特定的操作,比如点击、提交等。一些常用的WebElement方法包括:
Command | Description | Syntax |
---|---|---|
findElement() | This method finds the first element within the current web page by using given locator. | WebElement element = driverObject.findElement(By.locator(“value”)); |
sendKeys() | This method enters a value in to an Edit Box or Text box. | driver.findElement(By.elementLocator(“value”)).sendkeys(“value”); |
clear() | It clears the Value from an Edit box or Text Box. | driverObject.findElement(By.locatorname(“value”)).clear(); |
click() | It clicks an Element (Button, Link, Checkbox) etc. | driverObject.findElement(By.ElementLocator(“LocatorValue”)).click(); |
Selenium WebDriver 示例 – 打印网站标题
让我们来看一个使用Selenium WebDriver调用Firefox浏览器并打印网站标题的简单示例。
package com.Olivia.selenium.firefox;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class GeckoDriverExample {
public static void main(String[] args) {
//specify the location of GeckoDriver for Firefox browser automation
System.setProperty("webdriver.gecko.driver", "geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("https://scdev.com");
String PageTitle = driver.getTitle();
System.out.println("Page Title is:" + PageTitle);
driver.close();
}
}
输出:
1551941763563 mozrunner::runner INFO Running command: "/Applications/Firefox.app/Contents/MacOS/firefox-bin" "-marionette" "-foreground" "-no-remote" "-profile" "/var/folders/1t/sx2jbcl534z88byy78_36ykr0000gn/T/rust_mozprofile.t6ZyMHsrf2bh"
1551941764296 addons.webextension.screenshots@mozilla.org WARN Loading extension 'screenshots@mozilla.org': Reading manifest: Invalid host permission: resource://pdf.js/
1551941764297 addons.webextension.screenshots@mozilla.org WARN Loading extension 'screenshots@mozilla.org': Reading manifest: Invalid host permission: about:reader*
Can't find symbol 'GetGraphicsResetStatus'.
1551941765794 Marionette INFO Listening on port 61417
1551941765818 Marionette WARN TLS certificate errors will be ignored for this session
Mar 07, 2019 12:26:05 PM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Detected dialect: W3C
Page Title is:JournalDev - Java, Java EE, Android, Python, Web Development Tutorials
1551941814652 Marionette INFO Stopped listening on port 61417
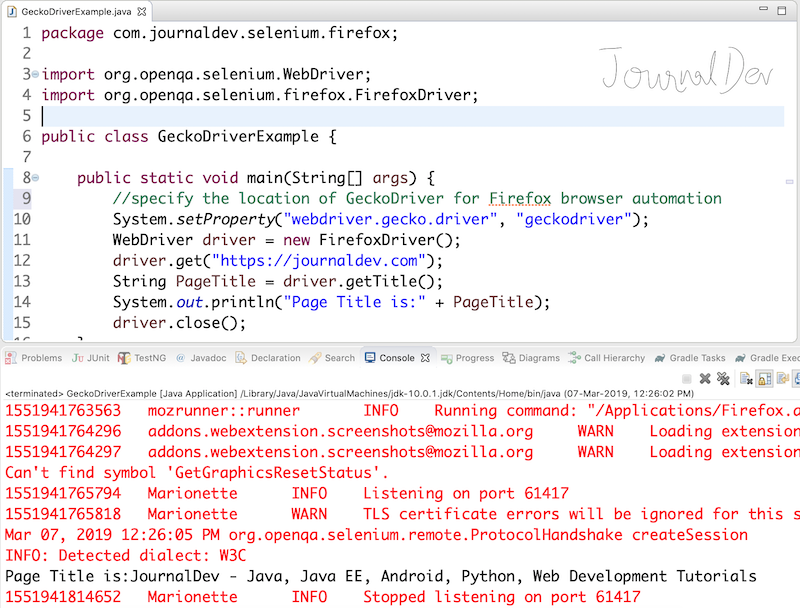
你可以从我们的GitHub存储库中查看更多的Selenium示例。
参考:WebDriver GitHub 代码